c#格式化输出console
时间: 2023-11-22 09:44:56 浏览: 206
C#中的格式化输出可以使用ToString()方法或者Console.WriteLine()方法。其中,ToString()方法可以对数字、日期等类型进行格式化输出,而Console.WriteLine()方法可以对字符串、数字等类型进行格式化输出。
下面是一些常用的格式化输出示例:
1. 对数字进行格式化输出:
double d = 12345678.2334;
Console.WriteLine(d.ToString("F2")); // 输出:12345678.23
Console.WriteLine(d.ToString("###,###.00")); // 输出:12,345,678.23
2. 对日期进行格式化输出:
DateTime dt = DateTime.Now;
Console.WriteLine(dt.ToString("yyyy-MM-dd")); // 输出:2021-08-10
Console.WriteLine(dt.ToString("yyyy年MM月dd日")); // 输出:2021年08月10日
3. 对字符串进行格式化输出:
string str = "hello";
Console.WriteLine("{0,-10}", str); // 输出:hello (左对齐)
Console.WriteLine("{0,10}", str); // 输出: hello(右对齐)
相关问题
c# 格式化输出字符串
在 C# 中,可以使用 `string.Format()` 方法来格式化输出字符串。该方法使用类似占位符的方式来指定输出字符串中的特定部分。以下是一个简单的例子:
```csharp
int num = 10;
string name = "John";
double price = 12.5;
string output = string.Format("The number is {0}, the name is {1}, and the price is {2:C}.", num, name, price);
Console.WriteLine(output);
```
输出结果为:
```
The number is 10, the name is John, and the price is $12.50.
```
在上面的代码中,`{0}`、`{1}` 和 `{2}` 是占位符,分别表示参数列表中的第一个、第二个和第三个参数。冒号后面的 `C` 表示将 `price` 参数格式化为货币形式。
除了上述示例中的格式化方式外,还有其他一些常用的格式化选项,例如日期时间格式化、数字格式化等等。您可以参考 C# 文档了解更多信息。
C#格式化输出二进制
在C#中,格式化输出二进制的方式可以通过使用位运算符和字符串操作来手动实现,但更简便的方法是利用内置的函数或者方法来转换。
一个简单的方法是使用`Convert.ToString`方法,它允许将整数转换为其二进制表示形式的字符串。可以通过指定基数(Base)参数为2来得到二进制格式的字符串。下面是一个简单的示例代码:
```csharp
int number = 123; // 这是一个十进制数
string binaryString = Convert.ToString(number, 2);
Console.WriteLine(binaryString); // 输出:1111011
```
在这个例子中,`Convert.ToString(number, 2)`将十进制数`123`转换为了其对应的二进制字符串`"1111011"`。
此外,C# 7.0引入了数字分隔符,可以让你在数字中加入下划线来提高可读性,但这些分隔符在字符串转换过程中会被忽略。例如:
```csharp
int number = 123;
string binaryString = Convert.ToString(number, 2);
Console.WriteLine($"The binary representation of {number} is {binaryString}"); // 输出:The binary representation of 123 is 1111011
```
阅读全文
相关推荐
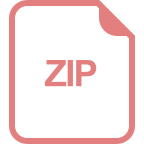
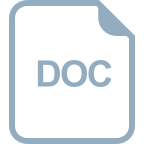
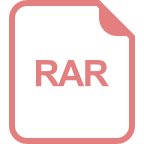
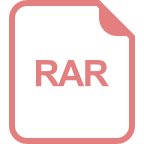
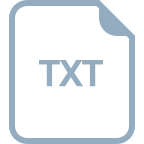
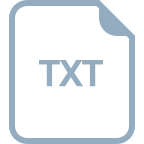
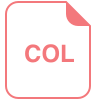
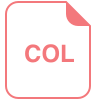






