C语言如何返回头指针
时间: 2024-10-21 20:10:25 浏览: 30
在C语言中,特别是涉及到数据结构如链表、树等时,我们常常需要创建并返回一个新的头指针来表示数据结构的起始位置。例如,如果你正在编写一个函数来创建一个新的单链表,那么这个函数可能会创建一个新的链表节点作为头结点,并通过返回值将这个头指针传递给调用者。
假设你有一个名为`create_linked_list()`的函数,它接收一组元素,然后创建一个包含这些元素的新链表:
```c
#include <stdlib.h> // 包含malloc和free的头文件
// 链表节点结构体
typedef struct Node {
int data; // 数据域
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建并返回链表的头指针
Node* create_linked_list(int *elements, size_t length) {
// 分配内存
Node* head = (Node*) malloc(sizeof(Node)); // 假设第一个节点总是第一个元素
if (!head) {
printf("Memory allocation failed.\n");
return NULL;
}
head->data = elements[0]; // 设置头节点的数据
head->next = NULL;
// 如果还有其他元素,递归地处理剩余部分
if (length > 1) {
Node* current = head;
for (size_t i = 1; i < length; i++) {
Node* newNode = (Node*) malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed at index %zu.\n", i);
break;
}
newNode->data = elements[i];
newNode->next = current->next;
current->next = newNode;
current = newNode; // 更新current以便于下一次迭代
}
}
return head;
}
// 主函数示例
int main() {
int numbers[] = {1, 2, 3, 4, 5};
size_t length = sizeof(numbers) / sizeof(numbers[0]);
Node* listHead = create_linked_list(numbers, length);
// 现在你可以用listHead操作你的链表...
free(listHead); // 别忘了释放内存
return 0;
}
```
在这段代码里,`create_linked_list()`函数首先创建一个头节点,然后根据输入数组`elements`的长度循环添加更多节点。最后,函数返回创建好的链表的头指针。注意,在使用完链表后,别忘了用`free()`释放头节点及其所有后续节点所占用的内存。
阅读全文
相关推荐
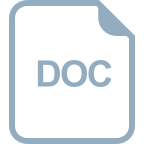
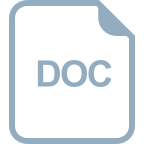
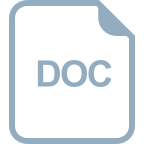
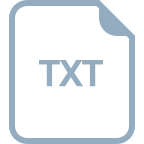
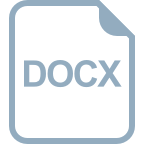
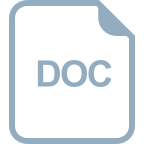
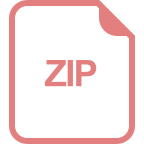
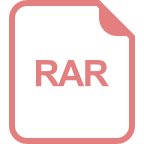
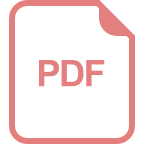