全部用python写出代码
时间: 2024-09-06 12:06:21 浏览: 33
为了用 Python 完成这个任务,我们需要导入必要的库并编写代码来实现上述的数学模型。这里是一个简化版的例子,假设数据存储在一个名为 `data` 的字典中,包含了作物列表、预期销售量、产量、成本和剩余作物处置价值。我们将使用 `pandas` 库来处理数据和创建 DataFrame,`numpy` 库用于数学运算,以及 `scipy.optimize` 进行线性规划。
```python
import pandas as pd
import numpy as np
from scipy.optimize import linprog
# 假设 data 数据结构如下:
# data = {
# 'crop_names': ['crop1', 'crop2', ...], # 作物名称
# 'sales': [expected_sales_1, expected_sales_2, ...], # 预期销量
# 'yield_per_acre': [yield_1, yield_2, ...], # 每亩产量
# 'cost_per_acre': [cost_1, cost_2, ...], # 每亩成本
# 'waste_value': waste_value, # 剩余作物价值(滞销)
# 'discount_rate': discount_rate, # 折价率
# }
# 创建 DataFrame
df = pd.DataFrame({
'Crop': data['crop_names'],
'Sales': data['sales'],
'Production': data['yield_per_acre'],
'Cost': data['cost_per_acre']
})
# 1. 模型1 (滞销情况)
def optimize_model1(df, waste_value):
production_matrix = df['Production'].values.reshape(-1, 1)
sales_vector = df['Sales'].values.reshape(-1, 1)
objective_function = -np.array([-df['Cost'].values]) # 目标函数 - 成本
constraints = [{'type': 'ineq', 'fun': lambda x: np.sum(x) - sales_vector - waste_value * np.sum(x)}]
bounds = [(0, None) for _ in range(len(df))]
res = linprog(objective_function, A_ub=production_matrix, b_ub=sales_vector.flatten(), bounds=bounds)
return res
# 2. 模型2 (降价出售情况)
def optimize_model2(df, discount_rate):
production_matrix = df['Production'].values.reshape(-1, 1)
sales_vector = df['Sales'].values.reshape(-1, 1)
objective_function = sales_vector - np.array([df['Cost'].values]) # 目标函数 - 成本
discounted_price = sales_vector * (1 - discount_rate)
constraints = [{'type': 'ineq', 'fun': lambda x: np.sum(x) - sales_vector}]
bounds = [(0, None) for _ in range(len(df))]
res = linprog(objective_function, A_ub=production_matrix, b_ub=discounted_price.flatten(), bounds=bounds)
return res
# 实际运行模型并保存结果到 Excel
result1 = optimize_model1(df, data['waste_value'])
result1_df = pd.DataFrame(result1.x, columns=['Quantity'])
result1_df.insert(0, 'Crop', data['crop_names'])
result2 = optimize_model2(df, data['discount_rate'])
result2_df = pd.DataFrame(result2.x, columns=['Quantity'])
result2_df.insert(0, 'Crop', data['crop_names'])
# 导出到 Excel 文件
with pd.ExcelWriter('result1.xlsx') as writer1:
result1_df.to_excel(writer1, sheet_name='Sheet1')
with pd.ExcelWriter('result2.xlsx') as writer2:
result2_df.to_excel(writer2, sheet_name='Sheet1')
```
这只是一个基本的示例,实际操作可能会更复杂,例如检查约束的有效性、异常处理等。请根据你的实际情况调整数据格式和文件路径。如果你有其他问题,比如数据预处理、Excel 文件操作或特定数学函数的理解,随时问我哦。
阅读全文
相关推荐
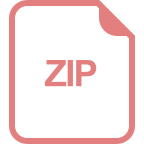
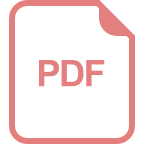
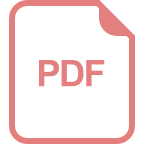















