十个八位密码Python
时间: 2024-05-13 20:11:34 浏览: 22
对于十个八位密码,我们可以使用Python中的随机数生成器来生成随机密码。以下是一个简单的Python代码示例,可以生成十个不同的八位密码:
```python
import random
import string
# 生成一个8位密码
def generate_password():
password = ''
for i in range(8):
# 从大小写字母和数字中随机选取一个字符
password += random.choice(string.ascii_letters + string.digits)
return password
# 生成十个不同的8位密码
passwords = set()
while len(passwords) < 10:
passwords.add(generate_password())
# 打印结果
for password in passwords:
print(password)
```
这段代码会使用Python的随机数生成器,从大小写字母和数字中随机选取一个字符,然后循环8次,将选取的字符组合成一个8位密码。接着,使用Python中的集合数据结构(set)来存储生成的密码,确保十个密码都是不同的。最后,打印出这十个不同的八位密码。
相关问题
26个大小写字母和10个数字随机生成十个八位密码Python
好的,以下是生成十个八位密码的 Python 代码:
```python
import random
import string
for i in range(10):
password = ''.join(random.choices(string.ascii_letters + string.digits, k=8))
print(password)
```
这段代码使用了 Python 内置的 `random` 模块和 `string` 模块。首先,我们将大小写字母和数字拼接起来,形成一个包含 62 个字符的字符串。然后,我们使用 `random.choices()` 函数从中随机选择 8 个字符,形成一个字符串作为密码。最后,我们使用 `print()` 函数将生成的密码打印出来。我们将这个过程重复执行 10 次,就可以得到 10 个随机生成的八位密码。
python随机生成十个八位密码
好的,这个问题很简单。可以使用Python的random模块和string模块来生成随机密码。以下是代码示例:
```python
import random
import string
# 定义密码长度
password_length = 8
# 定义密码数量
password_count = 10
# 定义密码字符集
password_characters = string.ascii_letters + string.digits
# 生成密码
for i in range(password_count):
password = ''.join(random.choice(password_characters) for i in range(password_length))
print(password)
```
这段代码将随机生成10个包含8个字符的密码,每个密码由数字和字母组成。
相关推荐
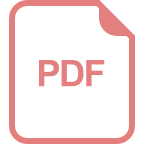












