Python 吸附窗口
时间: 2024-05-24 10:09:25 浏览: 15
Python 的吸附窗口指的是可以将窗口自动吸附到屏幕边缘或其他窗口边缘的功能。实现这个功能的主要方式是通过使用第三方库如 PyWin32 或 wxPython 等来访问操作系统的窗口管理 API,然后编写 Python 代码来实现吸附功能。
具体来说,实现吸附窗口的关键是要获取窗口的位置和大小信息,然后根据鼠标位置和窗口大小计算出吸附的位置。另外,还需要监听窗口移动和改变大小等事件,以便在窗口发生变化时重新计算吸附位置。
以下是一个使用 wxPython 实现窗口吸附功能的示例代码:
```python
import wx
class MyFrame(wx.Frame):
def __init__(self):
wx.Frame.__init__(self, None, title="My Frame", size=(300, 200))
self.Bind(wx.EVT_MOVE, self.OnMove)
self.Bind(wx.EVT_SIZE, self.OnSize)
def OnMove(self, event):
self.AdjustPosition()
def OnSize(self, event):
self.AdjustPosition()
def AdjustPosition(self):
screen_size = wx.GetDisplaySize()
self_size = self.GetSize()
self_pos = self.GetPosition()
# 判断是否需要吸附到屏幕边缘
if self_pos.x < 0:
self_pos.x = 0
elif self_pos.x + self_size.width > screen_size.width:
self_pos.x = screen_size.width - self_size.width
if self_pos.y < 0:
self_pos.y = 0
elif self_pos.y + self_size.height > screen_size.height:
self_pos.y = screen_size.height - self_size.height
# 判断是否需要吸附到其他窗口边缘
for other in wx.GetTopLevelWindows():
if other == self:
continue
other_pos = other.GetPosition()
other_size = other.GetSize()
if other_pos.x + other_size.width - self_size.width / 2 <= self_pos.x <= other_pos.x + other_size.width + self_size.width / 2 and \
other_pos.y + other_size.height - self_size.height / 2 <= self_pos.y <= other_pos.y + other_size.height + self_size.height / 2:
if abs(other_pos.x - self_pos.x) < abs(other_pos.y - self_pos.y):
if abs(self_pos.x + self_size.width - other_pos.x) < abs(self_pos.x - other_pos.x - other_size.width):
self_pos.x = other_pos.x - self_size.width
else:
self_pos.x = other_pos.x + other_size.width
else:
if abs(self_pos.y + self_size.height - other_pos.y) < abs(self_pos.y - other_pos.y - other_size.height):
self_pos.y = other_pos.y - self_size.height
else:
self_pos.y = other_pos.y + other_size.height
# 更新窗口位置
self.SetPosition(self_pos)
if __name__ == '__main__':
app = wx.App()
frame = MyFrame()
frame.Show(True)
app.MainLoop()
```
相关推荐
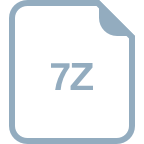














