帮我用vs做一个人事信息管理系统,并给出代码
时间: 2024-10-01 08:02:38 浏览: 27
创建一个人事信息管理系统通常涉及几个步骤,包括数据库设计、用户界面和业务逻辑处理。在这里,我将提供一个简单的概念框架和代码示例,以说明如何使用Visual Studio(VS)创建这样的系统。我们将使用C#和ASP.NET MVC作为开发平台。
首先,你需要安装Visual Studio并选择一个新的Web应用程序项目。以下是基本的步骤:
1. **模型(Model):**
```csharp
public class Employee
{
public int Id { get; set; }
public string Name { get; set; }
public string Position { get; set; }
// 添加其他字段如Email, DateOfBirth等
}
```
2. **数据访问层(DAL) - 连接数据库:**
使用Entity Framework或ADO.NET连接到数据库存储员工信息。这里仅展示基础设置,实际操作需要数据库连接字符串。
```csharp
using Microsoft.EntityFrameworkCore;
public class ApplicationDbContext : DbContext
{
public DbSet<Employee> Employees { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseSqlServer("your_connection_string");
}
}
```
3. **控制器(Controller):**
创建一个Controller来处理CRUD操作,如添加、编辑、删除和查看员工信息。
```csharp
[HttpGet("{id}")]
public ActionResult<Employee> GetEmployee(int id)
{
return await _context.Employees.FindAsync(id);
}
[HttpPost]
public ActionResult Create(Employee employee)
{
_context.Add(employee);
try
{
await _context.SaveChangesAsync();
}
catch (DbUpdateException ex)
{
// Handle exception
}
return RedirectToAction("Index");
}
```
4. **视图(View):**
使用Razor Pages或MVC视图来显示表单和列表,例如显示员工列表和编辑页面。
```html
@model IEnumerable<Employee>
<table>
<tr>
<th>Name</th>
<th>Position</th>
<!-- 添加更多列 -->
</tr>
@foreach (var employee in Model)
{
<tr>
<td>@employee.Name</td>
<td>@employee.Position</td>
<!-- 显示更多属性 -->
</tr>
}
</table>
```
这只是基本的框架,实际上可能还需要身份验证、错误处理、分页等功能。
阅读全文
相关推荐
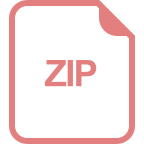
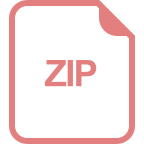
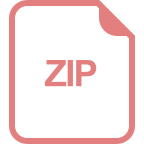
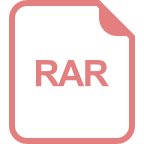
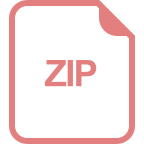
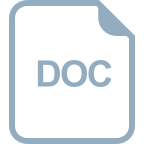
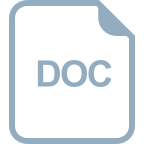
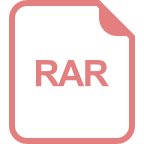
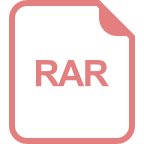
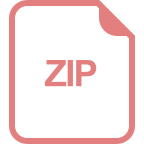
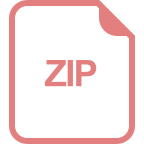
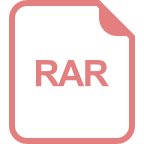
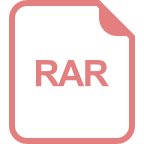
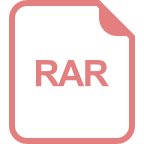
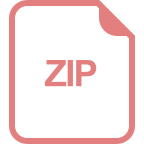
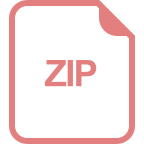
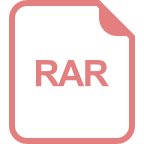