wpf中textbox只能输出string类型吗
时间: 2023-09-07 09:03:03 浏览: 186
在WPF中,TextBox控件的Text属性确实只能输出和接受string类型的数据。这是因为TextBox的主要用途是用于显示和编辑文本内容,而文本的基本数据类型就是字符串。
如果想要在TextBox中输出其他类型的数据,我们可以使用ToString()方法将其他类型的数据转换为字符串类型,然后将其赋值给TextBox的Text属性。例如,如果想要在TextBox中显示一个整数类型的数据,可以使用ToString()方法进行转换如下:
int number = 10;
textBox.Text = number.ToString();
或者可以使用字符串插值的方式将其他类型的数据转换为字符串后输出:
int number = 10;
textBox.Text = $"{number}";
当然,在从TextBox中获取值时,我们也可以将其转换为其他类型。根据需要,可以使用各种类型转换方法(如int.Parse()、float.Parse()等)或者类型转换函数(如Convert类中的方法)来将TextBox的文本内容转换为期望的数据类型。但是需要注意,这些转换过程中可能会出现格式不正确或者无法转换的情况,需要进行适当的异常处理。
总而言之,虽然TextBox主要用于字符串类型的输入和输出,但是我们可以通过合适的数据类型转换方法将其他类型的数据与TextBox进行交互。
相关问题
WPF中TEXTBOX的使用
在WPF中,TextBox是一个常用的控件,用于显示和编辑文本。以下是TextBox的使用方法:
1. 在XAML中创建一个TextBox控件。例如:
```
<TextBox Name="myTextBox" Width="200" Height="30" Text="Hello, World!" />
```
2. 在代码中可以通过以下方式获取或设置文本框中的文本:
```
string text = myTextBox.Text; // 获取文本框中的文本
myTextBox.Text = "New text"; // 设置文本框中的文本
```
3. TextBox控件还有一些其他的属性可供使用,如水印文字、密码模式、只读模式等。例如:
```
<TextBox Name="myTextBox" Width="200" Height="30" Text="Enter your name here..."
IsReadOnly="True" IsEnabled="False" IsPassword="True" />
```
这里的IsReadOnly属性表示文本框为只读模式,IsEnabled属性表示文本框被禁用,IsPassword属性表示文本框中输入的文本会被掩盖。
4. 可以通过事件来处理文本框中的文本变化。例如,当用户输入文本时,可以通过TextChanged事件来响应:
```
myTextBox.TextChanged += MyTextBox_TextChanged;
private void MyTextBox_TextChanged(object sender, TextChangedEventArgs e)
{
// 处理文本框中的文本变化
}
```
以上就是WPF中TextBox控件的使用方法,希望能对你有所帮助。
wpf中textbox中TextChanged属性如何绑定事件
在WPF中,`TextBox`的`TextChanged`属性用于监听文本框内容的变化。为了绑定这个事件并执行特定的操作,通常使用数据绑定和事件委托。以下是具体的步骤:
1. **创建视图模型**(ViewModel):
在您的视图模型(ViewModel)中,可以添加一个字符串属性来存储文本框的内容,并定义一个委托方法来处理文本变化。例如:
```csharp
public class ViewModel
{
public string TextBoxText { get; set; }
public event EventHandler<TextChangedEventArgs> TextChanged;
public void HandleTextChanged()
{
if (TextChanged != null)
{
TextChanged(this, new TextChangedEventArgs(TextBoxText));
}
}
}
public class TextChangedEventArgs : EventArgs
{
public string NewText { get; set; }
}
```
2. **XAML中绑定TextBox和订阅事件**:
在XAML中,首先声明一个`TextBox`并绑定其`Text`属性到`ViewModel`的`TextBoxText`,然后订阅`TextChanged`事件:
```xml
<TextBox x:Name="textBox">
<i:Interaction.Triggers>
<i:EventTrigger EventName="TextChanged">
<local:HandleTextChangedCommand/>
</i:EventTrigger>
</i:Interaction.Triggers>
</TextBox>
<!-- 这里引入了本地自定义命令,假设它位于一个叫本地的命名空间 -->
<Style TargetType="TextBox">
<Setter Property="Text" Value="{Binding TextBoxText}"/>
</Style>
```
这里的`HandleTextChangedCommand`应该是一个实例化了`ActionCommand`的绑定,它会在`TextChanged`发生时触发`ViewModel`的`HandleTextChanged`方法。
3. **实现自定义命令**:
如果需要的话,可以创建一个自定义命令(如`ActionCommand`),以便更好地控制何时执行回调函数。例如:
```csharp
public static class Commands
{
public static readonly RoutedCommand HandleTextChangedCommand = new();
}
```
4. **在代码-behind中注册事件处理器**:
在`.xaml.cs`文件中,注册`ViewModel`的`TextChanged`事件处理:
```csharp
private ViewModel viewModel;
public MainWindow()
{
InitializeComponent();
viewModel = new ViewModel();
viewModel.TextChanged += ViewModel_TextChanged;
}
private void ViewModel_TextChanged(object sender, TextChangedEventArgs e)
{
viewModel.HandleTextChanged();
}
```
现在每次`TextBox`中的文本改变,`HandleTextChanged`方法就会被调用,执行相应逻辑。
阅读全文
相关推荐
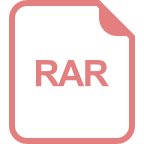
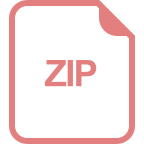
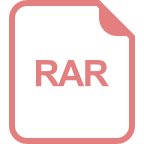


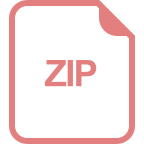









