使用mkfifo函数创建有名管道,并基于该管道实现两个进程间的通信。
时间: 2024-10-08 18:23:32 浏览: 60
在C语言中,`mkfifo()`函数用于在指定目录下创建一个命名管道(FIFO),也称为无名管道的一种特殊形式。这种管道是在文件系统中创建的一个特殊类型的文件,通常用于具有单个写者和多个读者的进程间通信。
为了基于这个有名管道实现两个进程之间的通信,你可以按照以下步骤操作:
1. **创建管道**:
使用`mkfifo()`函数创建一个 FIFO 文件,比如 `mypipe`. 例如:
```c
#include <unistd.h>
#include <fcntl.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <path_to_fifo>\n", argv[0]);
return -1;
}
const char *pipe_path = argv[1];
int result = mkfifo(pipe_path, S_IRUSR | S_IWUSR); // 设置权限,只允许所有者读写
if (result == -1) {
perror("Failed to create FIFO");
return -1;
}
printf("Named pipe created at %s\n", pipe_path);
return 0;
}
```
运行时传入你想创建管道的路径,如 `./create_pipe mypipe`.
2. **打开并监听管道**:
创建父进程,它将打开管道进行读取或写入。例如,父进程可能读取数据:
```c
parent_process.c
```
```c
#include <stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int read_from_fifo(const char *pipe_path) {
int fd = open(pipe_path, O_RDONLY); // 以只读模式打开
if (fd == -1) {
perror("Failed to open pipe");
return -1;
}
char buffer[100];
ssize_t bytes_read = read(fd, buffer, sizeof(buffer));
if (bytes_read == -1) {
perror("Failed to read from pipe");
} else {
printf("Received message: %.*s\n", bytes_read, buffer);
}
close(fd);
return 0;
}
int main() {
...
read_from_fifo("mypipe");
}
```
3. **创建子进程**:
子进程负责向管道写入数据:
```c
child_process.c
```
```c
#include <stdio.h>
#include <string.h>
void write_to_fifo(const char *pipe_path, const char *message) {
int fd = open(pipe_path, O_WRONLY); // 以只写模式打开
if (fd == -1) {
perror("Failed to open pipe for writing");
return;
}
size_t message_length = strlen(message);
if (write(fd, message, message_length) != message_length) {
perror("Failed to write to pipe");
}
close(fd);
}
int main() {
...
char msg[] = "Hello from child process!";
write_to_fifo("mypipe", msg);
}
```
运行这两个程序,先确保父进程运行,然后启动子进程。这样,子进程写入的数据就会被父进程读取,实现了进程间的通信。
阅读全文
相关推荐
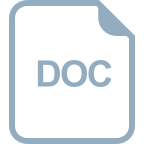
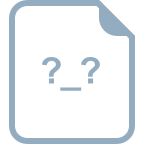
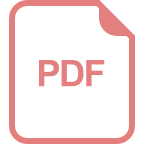

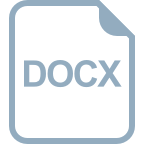
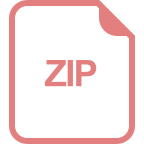
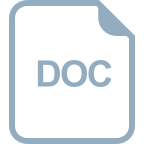
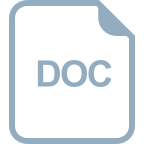
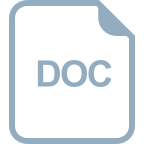
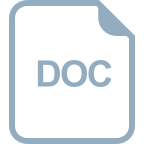
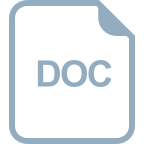
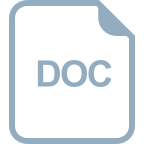
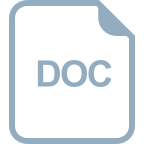
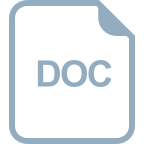
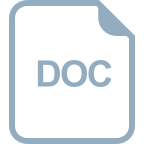
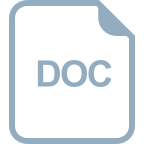
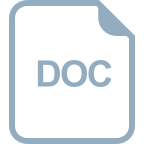
