//获取文件名字后缀 let file_ext = file.originalname.subString(file.originalname.lastIndexOf(".") + 1)
时间: 2024-04-12 07:29:45 浏览: 28
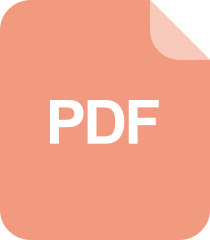
vue.js实现会动的简历(包含底部导航功能,编辑功能)
你可以使用以下代码获取文件名的后缀:
```javascript
let file_ext = file.originalname.substring(file.originalname.lastIndexOf(".") + 1);
```
这段代码通过找到最后一个点的位置,然后使用 `substring` 方法获取点后面的部分,即文件名的后缀。注意,这里使用的是 `substring` 方法而不是 `subString`。
阅读全文
相关推荐
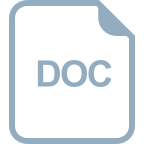
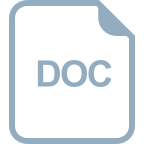

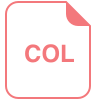
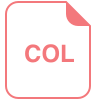




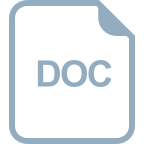
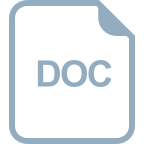
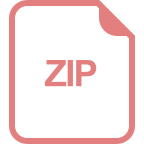
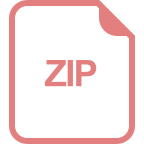
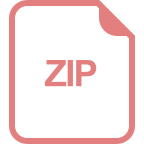