定义类并编写字符界面的Java Application程序,输入两个复数的实部和虚部,输出两个复数的和、差、积、商。
时间: 2024-10-17 15:13:19 浏览: 53
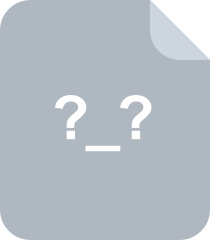
java 复数的类Complex

在Java中,你可以创建一个`ComplexNumber`类来表示复数,并提供相关的操作方法,如加法、减法、乘法和除法。以下是一个简单的示例:
```java
import java.util.Scanner;
class ComplexNumber {
double real;
double imaginary;
public ComplexNumber(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
// 加法
public ComplexNumber add(ComplexNumber other) {
return new ComplexNumber(this.real + other.real, this.imaginary + other.imaginary);
}
// 减法
public ComplexNumber subtract(ComplexNumber other) {
return new ComplexNumber(this.real - other.real, this.imaginary - other.imaginary);
}
// 积
public ComplexNumber multiply(ComplexNumber other) {
double realProduct = this.real * other.real - this.imaginary * other.imaginary;
double imagProduct = this.real * other.imaginary + this.imaginary * other.real;
return new ComplexNumber(realProduct, imagProduct);
}
// 商,需要检查除数是否为0
public ComplexNumber divide(ComplexNumber other) throws ArithmeticException {
if (other.real == 0 && other.imaginary == 0) throw new ArithmeticException("Cannot divide by zero");
double denominator = Math.pow(other.real, 2) + Math.pow(other.imaginary, 2);
return new ComplexNumber((this.real * other.real + this.imaginary * other.imaginary) / denominator,
(this.imaginary * other.real - this.real * other.imaginary) / denominator);
}
}
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the first complex number (real and imaginary parts):");
double real1 = scanner.nextDouble();
double imag1 = scanner.nextDouble();
ComplexNumber num1 = new ComplexNumber(real1, imag1);
System.out.println("Enter the second complex number (real and imaginary parts):");
double real2 = scanner.nextDouble();
double imag2 = scanner.nextDouble();
ComplexNumber num2 = new ComplexNumber(real2, imag2);
ComplexNumber sum = num1.add(num2);
ComplexNumber difference = num1.subtract(num2);
ComplexNumber product = num1.multiply(num2);
try {
ComplexNumber quotient = num1.divide(num2);
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
System.out.println("Product: " + product);
System.out.println("Quotient: " + quotient);
} catch (ArithmeticException e) {
System.out.println(e.getMessage());
}
scanner.close();
}
}
```
当你运行这个程序时,它会提示用户输入两个复数的实部和虚部,然后计算并显示它们的和、差、积以及商(如果除数不为零)。如果你尝试除以零,程序会捕获`ArithmeticException`并给出相应的错误消息。
阅读全文
相关推荐
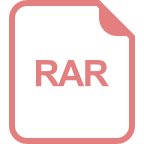
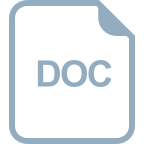



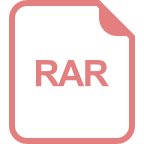
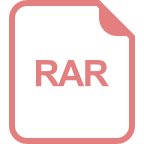
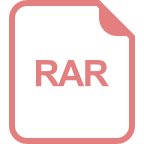
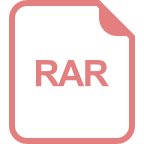
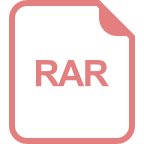
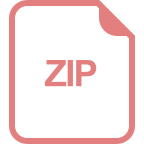
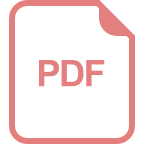
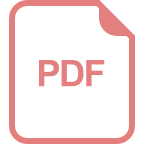
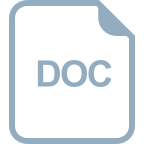
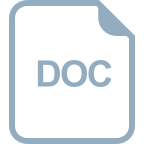
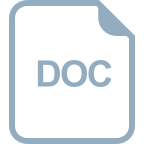
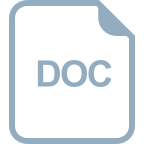
