用PYTHON写一个打印数据监控: ①监控打印服务器发送了打印数据, ②打印机是否接收到数据做监控,, ③打印机接收到打印指令后是否打印成功也做监控
时间: 2024-04-13 08:28:25 浏览: 203
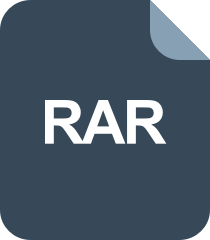
打印监控小工具,自己写的

以下是一个使用Python编写打印数据监控的示例代码,包括监控打印服务器是否发送了打印数据、打印机是否接收到数据以及打印机接收到指令后是否打印成功:
```python
import subprocess
import smtplib
from email.mime.text import MIMEText
from email.header import Header
# 监控函数
def monitor_printing():
# 打印服务器信息
printing_server_ip = 'your_printing_server_ip'
printing_server_log_path = 'your_printing_server_log_path'
# 打印机信息
printer_ip = 'your_printer_ip'
printer_status_command = 'lpstat -p'
# 邮件相关信息
smtp_server = 'your_smtp_server'
smtp_port = 587
smtp_username = 'your_smtp_username'
smtp_password = 'your_smtp_password'
sender_email = 'your_sender_email@example.com'
receiver_email = 'your_receiver_email@example.com'
subject = '打印数据监控报警'
# 检查打印服务器是否发送了打印数据
def check_printing_data():
try:
subprocess.run(['grep', '-r', 'Print job:', printing_server_log_path], check=True)
return True
except subprocess.CalledProcessError:
return False
# 检查打印机是否接收到数据
def check_printer_status():
try:
output = subprocess.check_output(printer_status_command, shell=True).decode('utf-8')
if printer_ip in output:
return True
else:
return False
except subprocess.CalledProcessError:
return False
# 发送邮件函数
def send_email(content):
message = MIMEText(content, 'plain', 'utf-8')
message['From'] = Header(sender_email)
message['To'] = Header(receiver_email)
message['Subject'] = Header(subject)
try:
smtp_obj = smtplib.SMTP(smtp_server, smtp_port)
smtp_obj.starttls()
smtp_obj.login(smtp_username, smtp_password)
smtp_obj.sendmail(sender_email, receiver_email, message.as_string())
smtp_obj.quit()
print('邮件已发送!')
except smtplib.SMTPException:
print('邮件发送失败!')
# 监控打印数据发送
if not check_printing_data():
content = '打印服务器未发送打印数据!'
send_email(content)
# 监控打印机接收数据和打印情况
if not check_printer_status():
content = '打印机未接收到数据或打印失败!'
send_email(content)
# 主函数
def main():
monitor_printing()
if __name__ == '__main__':
main()
```
请注意,您需要根据您的实际情况修改代码中的占位符 `'your_printing_server_ip'`,`'your_printing_server_log_path'`,`'your_printer_ip'`,`'your_smtp_server'`,`'your_smtp_username'`,`'your_smtp_password'`,`'your_sender_email@example.com'` 和 `'your_receiver_email@example.com'` 为实际的值。
此示例代码使用了 `subprocess` 模块来执行命令,`smtplib` 模块来发送邮件。监控函数中,通过检查打印服务器日志文件是否包含打印数据以及使用 `lpstat -p` 命令检查打印机状态来监控打印数据发送和打印机接收情况。如果有异常情况,会发送邮件进行报警。
希望对您有帮助!如有任何问题,请随时提问。
阅读全文
相关推荐
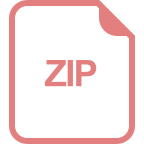
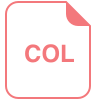
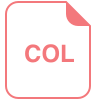
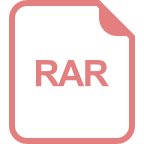
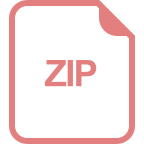
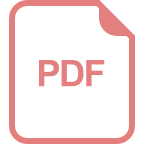
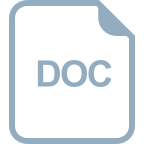
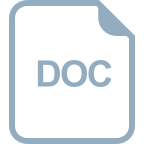
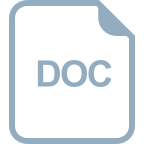
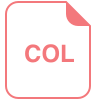
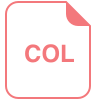
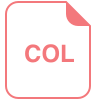
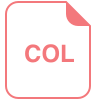
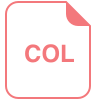
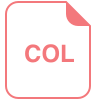
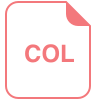
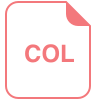