posix pthread
时间: 2023-08-27 08:04:43 浏览: 98
POSIX (Portable Operating System Interface) 是一个操作系统接口标准,定义了一组应用程序接口 (API),以便可移植地编写操作系统的应用程序。其中之一就是 POSIX 线程库(pthread),它提供了多线程编程的支持。
POSIX 线程库(pthread)是一套标准化的线程库,允许开发者在多线程环境中创建、同步和管理线程。它定义了一组用于线程创建、终止、同步、互斥、条件变量等操作的函数和数据类型。使用 pthread 库,可以方便地编写跨平台的多线程程序。
在 C/C++ 中使用 pthread 库时,需要包含头文件 `pthread.h`,并链接相应的库文件。然后可以使用 pthread 提供的函数来创建线程、进行线程同步等操作。
以下是一个简单的示例代码,展示了如何使用 pthread 库创建并运行两个线程:
```c
#include <stdio.h>
#include <pthread.h>
void* thread_func(void* arg) {
int thread_id = *(int*)arg;
printf("Thread %d is running\n", thread_id);
// 线程执行的代码
return NULL;
}
int main() {
pthread_t thread1, thread2;
int id1 = 1, id2 = 2;
// 创建线程
pthread_create(&thread1, NULL, thread_func, &id1);
pthread_create(&thread2, NULL, thread_func, &id2);
// 等待线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
return 0;
}
```
这个示例中,`thread_func` 函数是线程执行的代码,通过 `pthread_create` 创建两个线程,并通过 `pthread_join` 等待线程结束。注意,传递给线程函数的参数必须是指针类型。
这只是 pthread 库的基本用法,pthread 还提供了其他更多的函数和特性,如互斥锁、条件变量、线程属性等,可以根据具体需求进行使用。
阅读全文
相关推荐
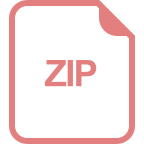
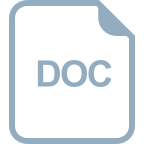
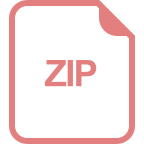
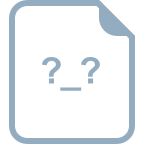
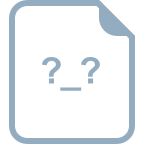
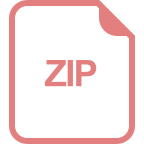
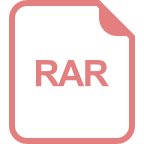
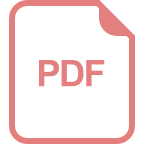
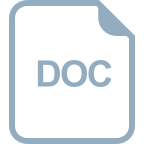
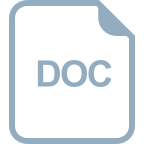
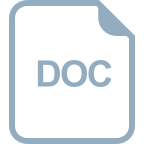
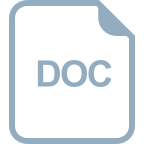

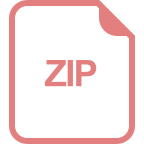
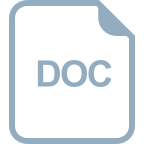
