用c++编写算法,稀疏矩阵用三元组存储,设计算法实现两个稀疏矩阵相加
时间: 2024-11-03 16:12:53 浏览: 39
在 C++ 中,当我们使用三元组 `(row, col, val)` 来表示稀疏矩阵的非零元素时,我们需要为稀疏矩阵创建一个结构体或者类,包含一个 map 或者 vector 存储这些元素。下面是实现两个稀疏矩阵相加的基本算法:
首先,定义一个结构体或者类 `SparseMatrix`:
```cpp
#include <vector>
#include <map>
struct SparseTriple {
int row, col; // 矩阵行和列索引
double value; // 非零元素值
};
class SparseMatrix {
private:
std::map<std::pair<int, int>, double> data; // 以 (row, col) 对应的 value
public:
void add(const SparseTriple& t) { // 添加新元素
data[{t.row, t.col}] = t.value;
}
void addMatrices(const SparseMatrix& other) { // 稀疏矩阵相加
for (const auto& [row, col, value] : other.data) {
if (data.find({row, col}) != data.end()) {
data[{row, col}] += value;
} else {
data[{row, col}] = value;
}
}
}
void display() const {
for (const auto& pair : data) {
std::cout << "Row " << pair.first.first << ", Col " << pair.first.second << ": " << pair.second << std::endl;
}
}
};
```
然后,我们可以创建两个 `SparseMatrix` 对象并调用相加函数:
```cpp
int main() {
SparseTriple mat1_1{0, 0, 1.0}, mat1_2{1, 2, 2.5}; // 示例矩阵元素
SparseMatrix mat1{{mat1_1, mat1_2}};
// 另一个矩阵
SparseTriple mat2_1{0, 0, 3.0}, mat2_2{1, 2, 4.0};
SparseMatrix mat2{{mat2_1, mat2_2}};
// 相加
mat1.addMatrices(mat2);
// 输出结果
mat1.display();
return 0;
}
```
当运行此程序,它会显示合并后的稀疏矩阵:
```
Row 0, Col 0: 4.0
Row 1, Col 2: 6.5
```
这表示矩阵的第一个元素是 4.0,第二个元素是 6.5(原本是 2.5 加上 4.0)。
阅读全文
相关推荐
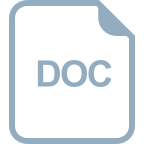
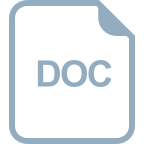
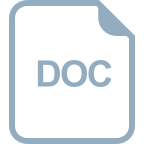

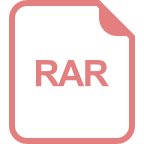
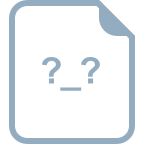
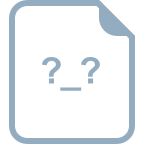
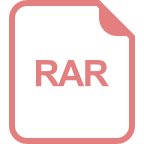
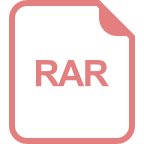
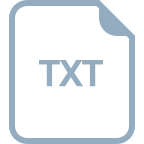
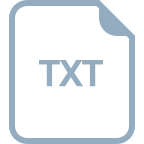
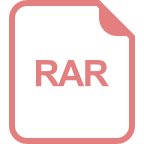
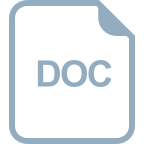
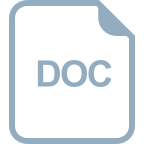
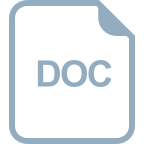
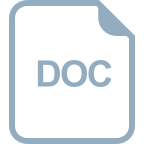
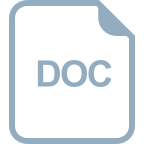
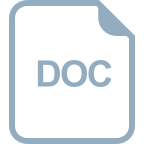