5、JSP页面中调用JavaBean有几种方法?分别是什么?
时间: 2024-10-11 10:04:34 浏览: 20
在JSP页面中,调用JavaBean主要有以下两种常见方法:
1. **隐式对象(Implicit Objects)**: JSP页面会自动创建一个名为`javaBeanName`的隐式对象,可以直接使用这个对象来访问JavaBean的属性和方法。例如:
```jsp
<jsp:useBean id="myBean" scope="session" class="com.example.MyBean"/>
${myBean.myProperty}
```
这里`<jsp:useBean>`标签定义了JavaBean,然后你可以通过`${}`表达式语法来读取属性。
2. **EL表达式(Expression Language)**: EL允许你在JSP页面上直接编写Java代码片段,包括对JavaBean的操作。例如:
```jsp
<bean:write property="myProperty" value="${myBean.myProperty}" />
<c:set var="myValue" value="${myBean.myMethod()}" />
```
`property`属性指定JavaBean的属性名,`value`属性则可以引用方法返回的结果。
相关问题
JSP页面中调用JavaBean有几种方法?分别是什么?
在JSP(JavaServer Pages)页面中调用JavaBean主要有以下两种方法:
1. **直接使用scriptlet标签**:在JSP文件中,你可以使用`<%=`标签直接引用JavaBean中的方法并执行,例如:
```jsp
<%@ page language="java" import="com.example.MyBean" %>
<%
MyBean myBean = new MyBean();
String result = myBean.myMethod();
out.println(result);
%>
```
这种方法直接嵌入了Java代码,不利于维护。
2. **使用EL表达式(Expression Language)**:从JSP 1.2开始,推荐使用EL来间接调用JavaBean的方法,使得代码更简洁,易于理解和维护:
```jsp
${myBean.myMethod()}
```
EL会解析为对MyBean实例的myMethod()方法的调用。
3. **利用JSTL标签库**:JSTL (JavaServer Pages Standard Tag Library) 提供了一些用于操作JavaBean的标签,如`<c:out>`标签结合EL可以方便地显示JavaBean的属性或调用方法:
```jsp
<c:out value="${myBean.myMethod()}"/>
```
jsp调用javabean封装Student类(包括no,name,age,gender等属性)、StudentTool类,分别用ArrayList和HashMap两种数据结构,实现学生信息的增删改查。
在JSP中调用JavaBean来操作`Student`类的信息,通常涉及到以下几个步骤:
1. **创建Student类**: 首先,你需要创建一个名为`Student`的JavaBean类,它包含no、name、age和gender等属性,例如:
```java
public class Student {
private int no;
private String name;
private int age;
private String gender;
// getters and setters...
}
```
2. **创建StudentTool类**: `StudentTool`类可以包含用于处理ArrayList和HashMap的数据操作方法。对于ArrayList:
```java
import java.util.ArrayList;
import java.util.List;
public class StudentTool {
private List<Student> studentList = new ArrayList<>();
public void add(Student s) {
studentList.add(s);
}
public boolean remove(int no) {
return studentList.removeIf(student -> student.getNo() == no);
}
// 提供其他方法如 update 和 find...
}
```
对于HashMap,你可以使用学生的ID作为键值对:
```java
import java.util.HashMap;
import java.util.Map;
public class StudentTool {
private Map<Integer, Student> studentMap = new HashMap<>();
public void add(Student s) {
studentMap.put(s.getNo(), s);
}
public boolean remove(int no) {
return studentMap.remove(no) != null;
}
// 提供get方法获取学生,update方法更新学生信息...
}
```
3. **在JSP中调用**: 在JSP文件中,你可以通过`<jsp:useBean>`标签实例化`StudentTool`类,并通过它的方法进行CRUD操作,比如:
```jsp
<%@ page import="com.example.Student, com.example.StudentTool" %>
...
<jsp:useBean id="studentTool" scope="session" class="com.example.StudentTool" />
...
<form action="yourAction">
<input type="text" name="no" ... />
<input type="submit" value="添加" onclick="addStudent()" />
</form>
<script>
function addStudent() {
var formData = ...; // 获取表单数据
var student = new Student(...);
studentTool.add(student);
// 后端处理并返回结果
}
</script>
```
阅读全文
相关推荐
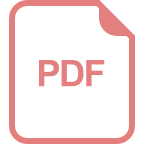
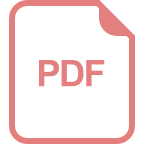
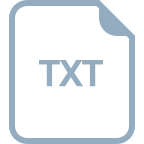
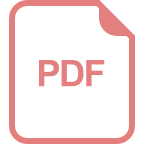
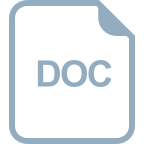
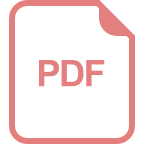
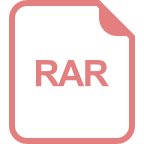
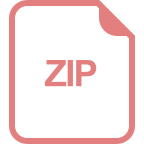
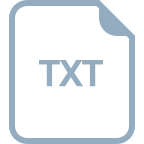
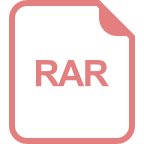
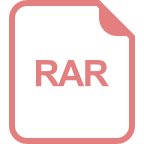
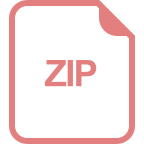
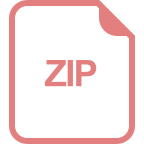
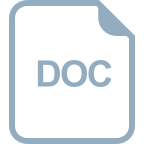
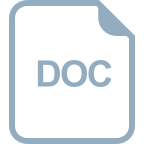
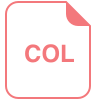
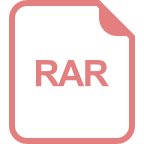