C语言输入多个字符串
时间: 2024-09-18 08:03:47 浏览: 27
在C语言中,通常通过循环结构和`scanf`函数或者`fgets`函数来输入多个字符串。这里有两个常用的方法:
1. **使用`scanf`和数组**:
```c
#include <stdio.h>
int main() {
char str[100][50]; // 创建一个二维字符数组存储字符串
int count = 0;
while (count < 10) { // 限制最多输入10个字符串
printf("请输入第 %d 个字符串:", count + 1);
scanf("%s", str[count++]); // 使用"%s"作为格式说明符读取字符串,注意要加上换行符"\n"
}
for (int i = 0; i < count; i++) {
printf("输入的字符串:%s\n", str[i]);
}
return 0;
}
```
2. **使用`fgets`和动态内存分配**:
```c
#include <stdio.h>
#include <stdlib.h> // 包含malloc和free函数
int main() {
int count = 0;
char** strings = malloc(sizeof(char*) * 10); // 预先分配空间给10个字符串指针
while (count < 10) {
char input[100];
fgets(input, sizeof(input), stdin); // 从标准输入读取一行
input[strcspn(input, "\n")] = '\0'; // 去除末尾的换行符
strings[count] = strdup(input); // 拷贝字符串并分配新的内存
count++;
}
// ...处理输入的字符串...
free(strings); // 释放动态分配的内存
return 0;
}
```
相关推荐
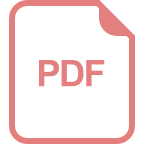
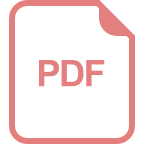
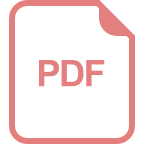
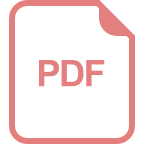
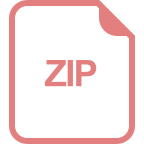
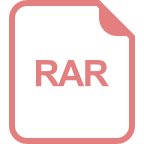
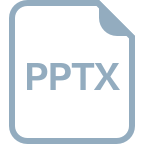
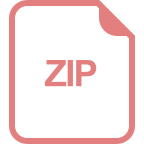
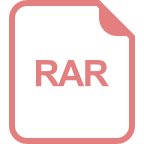
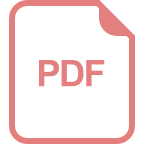
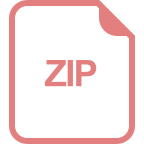
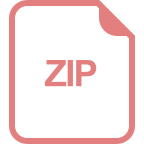
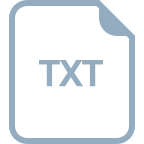
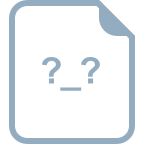
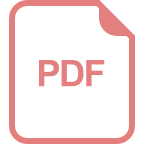
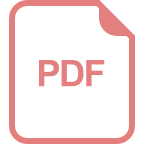
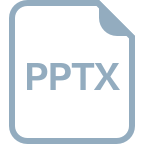
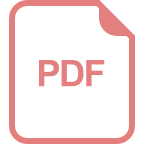