爬虫豆瓣电影类别获取代码
时间: 2023-12-17 22:18:52 浏览: 50
下面是一个使用Python的代码示例来爬取豆瓣电影的类别信息:
```python
import requests
from bs4 import BeautifulSoup
# 发起HTTP请求获取网页内容
url = 'https://movie.douban.com/chart'
response = requests.get(url)
html = response.text
# 解析网页内容
soup = BeautifulSoup(html, 'html.parser')
# 获取电影类别信息
category_list = soup.select('.types span')
categories = [category.text for category in category_list]
# 打印电影类别
for category in categories:
print(category)
```
这段代码使用了`requests`库来发起HTTP请求获取豆瓣电影排行榜页面的内容,然后使用`BeautifulSoup`库解析网页内容。通过选择器选取类名为`.types`下的`span`标签,获取电影类别信息并存储在`categories`列表中。最后,通过循环打印出所有的电影类别。
注意:请确保你已经安装了`requests`库和`beautifulsoup4`库。可以使用以下命令来安装:
```
pip install requests
pip install beautifulsoup4
```
相关问题
爬虫豆瓣电影类别代码
豆瓣电影的类别代码可以通过以下方式进行爬取:
```python
import requests
from bs4 import BeautifulSoup
url = "https://movie.douban.com/chart" # 豆瓣电影排行榜页面
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
category_list = soup.find_all("a", class_="tag") # 定位到类别标签
for category in category_list:
print(category.text)
```
上述代码使用了 `requests` 库发送请求,并使用 `BeautifulSoup` 库解析网页内容。通过指定类名为 `"tag"` 的 `<a>` 标签,可以获取到豆瓣电影的各个类别名称。
运行代码后,你将会得到豆瓣电影的类别列表。希望能对你有所帮助!如果有任何问题,请随时提问。
python用简单的selenium爬虫爬去豆瓣电影豆瓣电影排行榜前250部电影的电影名称 导演 主演 年份 国别 类别 评分 评价人数
好的,以下是代码实现:
```python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.keys import Keys
# 设置 ChromeDriver 路径
driver_path = 'chromedriver.exe'
# 初始化 ChromeDriver
driver = webdriver.Chrome(executable_path=driver_path)
# 打开豆瓣电影排行榜页面
driver.get('https://movie.douban.com/chart')
# 获取所有电影条目
movie_items = WebDriverWait(driver, 10).until(
EC.presence_of_all_elements_located((By.XPATH, '//div[@class="pl2"]'))
)
# 遍历电影条目,获取电影信息
for i, item in enumerate(movie_items[:250]):
print(f'#{i+1}')
# 获取电影名称
movie_name = item.find_element_by_tag_name('a').text
print(f'电影名称:{movie_name}')
# 进入电影详情页
item.find_element_by_tag_name('a').click()
# 获取电影信息
try:
# 获取导演、主演、年份、国别、类别、评分、评价人数
director = driver.find_element_by_xpath('//span[contains(text(),"导演")]/following-sibling::span[1]').text
actors = driver.find_element_by_xpath('//span[contains(text(),"主演")]/following-sibling::span[1]').text
year = driver.find_element_by_xpath('//span[contains(text(),"年份")]/following-sibling::span[1]').text
country = driver.find_element_by_xpath('//span[contains(text(),"制片国家")]/following-sibling::span[1]').text
genre = driver.find_element_by_xpath('//span[contains(text(),"类型")]/following-sibling::span[1]').text
rating = driver.find_element_by_xpath('//strong[contains(@class,"rating_num")][1]')\
.get_attribute('textContent')
rating_num = driver.find_element_by_xpath('//span[contains(@class,"rating_num")][1]')\
.get_attribute('textContent')
# 打印电影信息
print(f'导演:{director}')
print(f'主演:{actors}')
print(f'年份:{year}')
print(f'国别:{country}')
print(f'类别:{genre}')
print(f'评分:{rating}')
print(f'评价人数:{rating_num}')
except:
print('获取电影信息失败')
# 返回电影排行榜页面
driver.back()
# 刷新电影条目列表
movie_items = WebDriverWait(driver, 10).until(
EC.presence_of_all_elements_located((By.XPATH, '//div[@class="pl2"]'))
)
# 关闭浏览器
driver.quit()
```
需要注意的是,在获取导演、主演、年份、国别、类别、评分和评价人数时,需要使用 `get_attribute('textContent')` 来获取元素的文本内容。此外,由于豆瓣电影详情页获取电影信息的方式比较特殊,需要通过点击电影条目进入详情页,再返回电影排行榜页面,刷新电影条目列表的方式来实现遍历所有电影信息的目的。
相关推荐
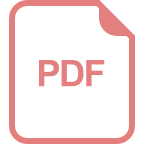
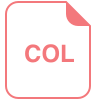








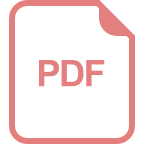