python绘图 ro bs
时间: 2023-10-30 17:00:58 浏览: 68
在Python中使用matplotlib库进行绘图时,可以使用不同的参数设置来调整绘图的形状和颜色。在引用中的示例中,"ro"代表红色的圆圈标记,而"bs"代表蓝色的方块标记。这些参数可以在plot函数中使用,例如:plt.plot(x, y, 'ro')表示在坐标(x, y)处绘制红色的圆圈标记。同样地,可以使用'bs'来代表蓝色的方块标记。绘图的具体形状和颜色可以根据需要进行调整。
相关问题
python绘图 sci
Python绘图可以应用于科学研究领域,特别是在SCI论文的绘图部分。Python提供了很多绘图工具和库,例如Matplotlib、Seaborn等,可以用于生成科学风格的图表。使用Python绘图可以实现对数据的可视化展示,方便观察数据的规律和趋势。
在进行Python绘图时,可以使用Jupyter Notebook作为“前处理”工具,用于初始生成图片。之后可以使用PPT对图片进行后期加工。对于分子动力学的数据分析及Python绘图,可以结合Matlab和Python来完成。当然,在绘制SCI论文图表时,可以根据个人喜好和相关期刊的风格进行绘图,绘图模板可以作为参考。
在处理相同数据图片时,可以使用Jupyter Notebook进行循环绘图,方便快捷。Python绘图的基本工具包括图片初始生成、图片处理的后期加工和针对分子动力学的数据分析及Python绘图。绘图程序模板包括输出图片文件储存、Excel文件读取和图片格式子函数等。
以下是关于Python绘图SCI论文的常见问题:
1. Python绘图在SCI论文中的应用有哪些特点?
2. 有没有一些常用的Python绘图模板可以分享?
3. Python绘图与其他绘图工具相比有什么优势?
python绘图汉化
对于Python绘图的汉化,可以使用turtle模块来实现。turtle模块是Python中的一个绘图库,提供了一些简单的绘图函数,可以用来绘制各种形状和图案。
要实现Python绘图的汉化,可以按照以下步骤进行操作:
1. 导入turtle模块:
```python
import turtle
```
2. 设置绘图窗口的标题:
```python
turtle.title("绘图汉化示例")
```
3. 设置绘图窗口的背景颜色:
```python
turtle.bgcolor("white")
```
4. 设置画笔的颜色:
```python
turtle.pencolor("black")
```
5. 设置画笔的粗细:
```python
turtle.pensize(2)
```
6. 绘制图形:
```python
turtle.forward(100) # 向前移动100个像素
turtle.right(90) # 向右转90度
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
```
7. 关闭绘图窗口:
```python
turtle.done()
```
通过以上步骤,就可以实现Python绘图的汉化效果了。
相关推荐
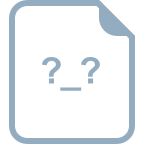












