AttributeError: 'numpy.ndarray' object has no attribute 'getA'
时间: 2023-11-17 09:07:04 浏览: 49
这个错误通常是因为你正在尝试使用NumPy数组上不存在的方法或属性。可能是因为你的代码中有一些错误,导致你的数组不是你以为的类型。你可以检查一下你的代码,确保你正在使用正确的方法和属性。如果你仍然无法解决问题,你可以尝试使用Python的内置调试器来查找问题所在。
以下是一个例子,展示了如何使用NumPy数组,并且不会出现'numpy.ndarray' object has no attribute 'getA'的错误:
```python
import numpy as np
# 创建一个2x2的数组
arr = np.array([[1, 2], [3, 4]])
# 输出数组的类型
print(type(arr)) # 输出:<class 'numpy.ndarray'>
# 输出数组的形状
print(arr.shape) # 输出:(2, 2)
# 输出数组的第一个元素
print(arr[0, 0]) # 输出:1
# 将数组转换为列表
lst = arr.tolist()
# 输出列表的类型
print(type(lst)) # 输出:<class 'list'>
# 输出列表的第一个元素
print(lst[0][0]) # 输出:1
```
相关问题
AttributeError: 'numpy.ndarray' object has no attribute 'numpy'
This error occurs when you try to access the `numpy` attribute of a `numpy.ndarray` object, but the attribute doesn't exist.
For example, consider the following code:
```python
import numpy as np
arr = np.array([1, 2, 3])
arr.numpy()
```
When you try to execute the `arr.numpy()` line, you'll get the `AttributeError` because there's no `numpy` attribute in the `ndarray` object.
To fix this error, you need to remove the `.numpy()` part of the code and simply use the `arr` object directly. For example:
```python
import numpy as np
arr = np.array([1, 2, 3])
print(arr)
```
This will print the contents of the `arr` object, which is `[1 2 3]`.
AttributeError: 'numpy.ndarray' object has no attribute 'get'
This error occurs when you try to access the "get" attribute of a NumPy array, but NumPy arrays do not have a "get" attribute.
It is possible that the code you are working with is written for a different type of object that does have a "get" attribute. You may need to review the code and ensure that it is compatible with NumPy arrays.
If you are trying to access a specific element or subset of elements within the array, you can use indexing or slicing to retrieve the desired values. For example:
```
import numpy as np
my_array = np.array([1, 2, 3, 4, 5])
print(my_array[2]) # Output: 3
print(my_array[1:4]) # Output: [2 3 4]
```
相关推荐
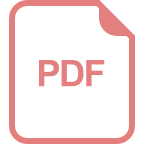
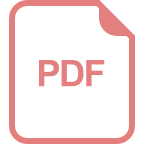
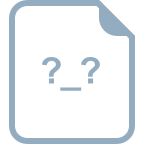
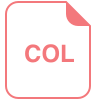











