springboot 串口通讯
时间: 2023-11-08 10:06:06 浏览: 96
可以使用Java的RXTX库来实现Spring Boot与串口通讯。首先需要在pom.xml文件中添加RXTX依赖:
```
<dependency>
<groupId>org.rxtx</groupId>
<artifactId>rxtx</artifactId>
<version>2.2</version>
</dependency>
```
然后在代码中使用SerialPort类来打开串口、读取数据和发送数据。以下是一个简单的示例:
```
import gnu.io.CommPortIdentifier;
import gnu.io.SerialPort;
import org.springframework.stereotype.Component;
import javax.annotation.PostConstruct;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
@Component
public class SerialPortService {
private static final String PORT_NAME = "/dev/ttyUSB0"; // 串口名称
private static final int BAUD_RATE = 9600; // 波特率
private SerialPort serialPort;
@PostConstruct
public void init() throws Exception {
CommPortIdentifier portIdentifier = CommPortIdentifier.getPortIdentifier(PORT_NAME);
if (portIdentifier.isCurrentlyOwned()) {
throw new Exception("Port is currently in use");
} else {
serialPort = (SerialPort) portIdentifier.open(this.getClass().getName(), 2000);
serialPort.setSerialPortParams(BAUD_RATE, SerialPort.DATABITS_8, SerialPort.STOPBITS_1, SerialPort.PARITY_NONE);
}
}
public void sendData(byte[] data) throws IOException {
OutputStream outputStream = serialPort.getOutputStream();
outputStream.write(data);
outputStream.flush();
}
public byte[] readData() throws IOException {
InputStream inputStream = serialPort.getInputStream();
byte[] buffer = new byte[1024];
int len = inputStream.read(buffer);
byte[] data = new byte[len];
System.arraycopy(buffer, 0, data, 0, len);
return data;
}
}
```
阅读全文
相关推荐








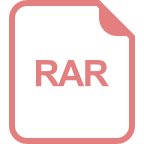



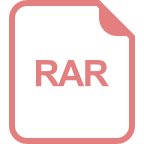
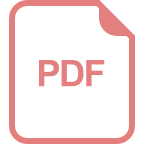




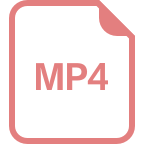