生成一个c++烟花代码
时间: 2024-10-14 09:04:17 浏览: 48
在C++中编写一个简单的烟花效果代码通常涉及到图形库如SFML、SDL或者是直接使用操作系统提供的API。由于这是一个相对复杂的任务,这里我会提供一个基本的控制台模拟烟花的简单示例,实际的图形化烟花效果会更复杂,涉及到像素操作和动画。
```cpp
#include <iostream>
#include <thread> // 引入线程库
// 简化的烟花效果类
class Firework {
public:
void launch(int x, int y) {
for (int i = 0; i < 5; ++i) {
std::cout << "Firework launched at (" << x << ", " << y << ") - explosion " << i+1 << "\n";
std::this_thread::sleep_for(std::chrono::milliseconds(100)); // 模拟烟花爆炸延迟
}
}
};
int main() {
Firework firework;
firework.launch(100, 100); // 在坐标(100, 100)处发射烟花
return 0;
}
相关问题
生成一个烟花代码c++
在C++中创建一个简单的烟花效果通常涉及到图形库,比如SFML、SDL或者直接使用Windows API。这里是一个非常基础的例子,使用C++和Windows API绘制点状烟花:
```cpp
#include <windows.h>
#include <iostream>
void drawFireworks(int x, int y, int radius) {
for (int i = 0; i < 50; ++i) {
POINT point;
point.x = x + rand() % radius * 2 - radius; // 随机位置在圆内
point.y = y + rand() % radius * 2 - radius;
SetPixel(hdc, point.x, point.y, RGB(255, 255, 255)); // 绘制白色像素
}
}
int main() {
HDC hdc = GetDC(NULL); // 获取设备上下文
HBRUSH brush = CreateSolidBrush(RGB(0, 0, 0)); // 创建黑色刷子,用于清除屏幕
for (int i = 0; i < 5; ++i) { // 循环绘制几次烟花
drawFireworks(i * 100, 200, 50); // 烟花中心位置和半径
Sleep(200); // 暂停200毫秒让烟花绽放
FillRect(hdc, &RECT{i*100, 200, i*100+50, 200+50}, brush); // 清除区域
}
ReleaseDC(NULL, hdc);
DeleteObject(brush);
return 0;
}
```
这个代码会在屏幕上随机绘制出多个小圆点,看起来类似烟花。请注意,这只是一个简化的示例,实际的烟花动画会更复杂,可能需要处理动画帧和颜色变化。
生成c++烟花代码
好的,以下是一个简单的C++烟花代码,可以在控制台中运行:
```c++
#include <iostream>
#include <windows.h>
#include <stdlib.h>
#include <time.h>
using namespace std;
void setColor(int color) {
SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), color);
}
void gotoxy(int x, int y) {
COORD pos = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void draw(int x, int y, char c, int color) {
setColor(color);
gotoxy(x, y);
cout << c;
}
int main() {
srand(time(NULL));
int screenWidth = 80;
int screenHeight = 40;
int numParticles = 100;
int gravity = 1;
int colors[] = {7, 9, 10, 11, 12, 13, 14};
while (true) {
// 初始化烟花粒子
int x = rand() % screenWidth;
int y = screenHeight - 1;
int vx = rand() % 6 - 3;
int vy = rand() % 6 - 10;
int color = colors[rand() % 7];
// 烟花爆炸
for (int i = 0; i < 15; i++) {
draw(x, y, '*', color);
x += vx;
y += vy;
vy += gravity;
Sleep(50);
}
// 烟花粒子消失
for (int i = 0; i < numParticles; i++) {
int px = x;
int py = y;
int pvx = rand() % 6 - 3;
int pvy = rand() % 6 - 3;
int pcolor = colors[rand() % 7];
for (int j = 0; j < 10; j++) {
draw(px, py, '.', pcolor);
px += pvx;
py += pvy;
pvy += gravity;
Sleep(50);
}
}
}
return 0;
}
```
这个代码会在控制台中不断产生烟花爆炸效果,每个烟花会分裂成多个粒子,最终逐渐消失。
阅读全文
相关推荐
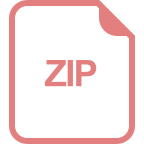
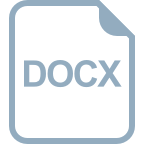
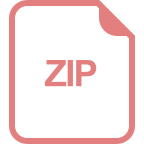











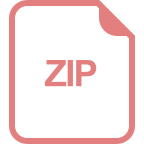
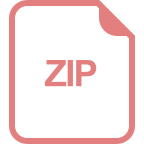
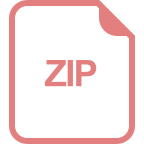