java http发送xml报文加上密码认证
时间: 2023-10-16 16:08:00 浏览: 54
您可以使用Java的HttpClient库来发送HTTP请求,并在请求中添加XML报文和密码认证。下面是一个示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpHeaders;
import org.apache.http.auth.AuthScope;
import org.apache.http.auth.UsernamePasswordCredentials;
import org.apache.http.client.CredentialsProvider;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.BasicCredentialsProvider;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
public class HttpClientExample {
public static void main(String[] args) {
// 设置用户名和密码
String username = "your_username";
String password = "your_password";
// 创建CredentialsProvider并设置用户名和密码
CredentialsProvider credentialsProvider = new BasicCredentialsProvider();
credentialsProvider.setCredentials(AuthScope.ANY, new UsernamePasswordCredentials(username, password));
// 创建HttpClient对象并设置CredentialsProvider
CloseableHttpClient httpClient = HttpClients.custom()
.setDefaultCredentialsProvider(credentialsProvider)
.build();
// 创建HttpPost对象
HttpPost httpPost = new HttpPost("http://example.com/api/endpoint");
// 设置请求头
httpPost.setHeader(HttpHeaders.CONTENT_TYPE, ContentType.APPLICATION_XML.getMimeType());
// 设置XML报文
String xmlPayload = "<xml>...</xml>";
httpPost.setEntity(new StringEntity(xmlPayload, ContentType.APPLICATION_XML));
try {
// 发送请求并获取响应
CloseableHttpResponse response = httpClient.execute(httpPost);
// 处理响应
HttpEntity entity = response.getEntity();
if (entity != null) {
String responseString = EntityUtils.toString(entity); System.out.println(responseString);
}
// 关闭响应和HttpClient
response.close();
httpClient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请替换示例代码中的`http://example.com/api/endpoint`为您要发送请求的实际URL,`<xml>...</xml>`为您要发送的XML报文内容。另外,将`your_username`和`your_password`替换为实际的用户名和密码。
相关推荐
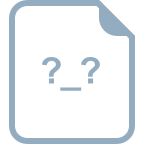
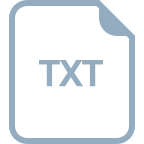














