c++线程池运行三个任务代码示例
时间: 2024-09-09 18:14:01 浏览: 28
在 C++ 中,可以使用 `std::thread_pool` 或第三方库如 C++17 引入的 `<execution>` 模块创建线程池。这里是一个简单的例子,展示如何使用 `std::ThreadPool` 运行三个任务:
```cpp
#include <iostream>
#include <thread>
#include <future>
#include <vector>
#include <thread_pool>
int add(int a, int b) {
return a + b;
}
void print_hello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
// 创建一个包含4个线程的线程池
std::ThreadPool executor(4);
// 准备要执行的任务
auto task1 = std::packaged_task<int(int, int)>(add);
auto task2 = std::packaged_task<void()> (print_hello);
auto task3 = []() { /* 这里可以编写任何函数体 */ };
// 将任务提交给线程池
auto future1 = executor.submit(task1, 5, 10);
auto future2 = executor.submit(std::move(task2));
executor.submit(std::move(task3));
// 等待所有任务完成
try {
int result1 = future1.get(); // 获取第一个任务的结果
std::cout << "Result of task 1: " << result1 << std::endl;
// 由于task2没有返回值,get()会直接执行
future2.get();
} catch (std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
在这个示例中,我们创建了一个线程池并提交了三个任务:两个有确定结果的任务(通过`std::packaged_task`),以及一个无返回值的任务。线程池会自动管理线程,当线程池满或者任务完成后,新任务才会被添加。
相关推荐
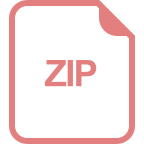
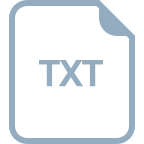















