C#作为客户端 读取http请求中的json格式数据
时间: 2024-09-09 15:08:20 浏览: 52
C#作为客户端读取HTTP请求中的JSON格式数据,通常是通过HTTP客户端类库来完成的。在.NET框架中,可以使用HttpClient类来发送HTTP请求并接收响应。如果响应的内容是JSON格式的数据,可以使用JSON解析库(如Newtonsoft.Json)来将JSON字符串转换为C#中的对象。
以下是使用HttpClient读取JSON数据并解析的基本步骤:
1. 创建HttpClient实例并配置请求。
2. 发送GET或POST等HTTP请求。
3. 接收响应内容,并确保其为JSON格式。
4. 使用JSON解析器将JSON字符串转换为C#对象。
下面是一个简单的示例代码:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
using Newtonsoft.Json;
public class JsonData
{
public string Property1 { get; set; }
public int Property2 { get; set; }
// 其他属性...
}
class Program
{
static async Task Main(string[] args)
{
using (var httpClient = new HttpClient())
{
try
{
// 发送GET请求
HttpResponseMessage response = await httpClient.GetAsync("http://example.com/api/data");
response.EnsureSuccessStatusCode(); // 确保响应成功
// 读取响应内容
string responseBody = await response.Content.ReadAsStringAsync();
// 解析JSON到对象
JsonData data = JsonConvert.DeserializeObject<JsonData>(responseBody);
// 使用data对象中的数据
Console.WriteLine($"Property1: {data.Property1}, Property2: {data.Property2}");
}
catch (HttpRequestException e)
{
Console.WriteLine("\nException Caught!");
Console.WriteLine("Message :{0} ", e.Message);
}
}
}
}
```
在上面的代码中,我们定义了一个名为JsonData的类,用于表示要解析的JSON数据的结构。然后通过HttpClient发送请求,并在接收到响应后将其内容读取为字符串。使用JsonConvert.DeserializeObject方法将字符串解析为JsonData对象。
阅读全文
相关推荐
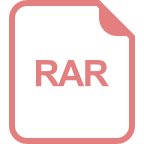
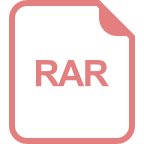
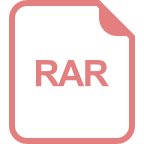
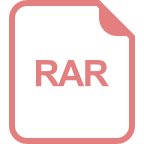
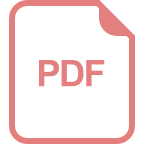
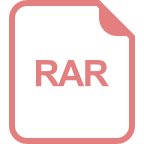
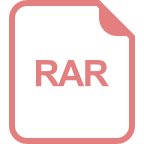
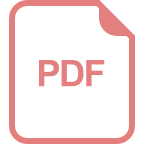
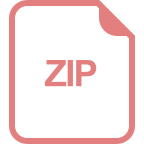
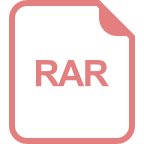
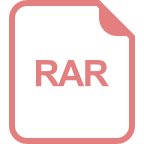
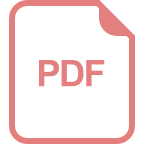
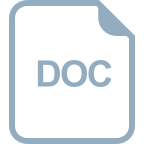
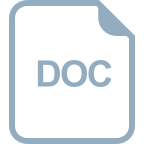
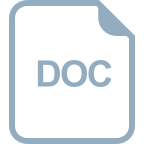
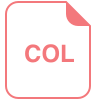

