输入圆的半径r,计算园周长和园面积,保留2位小数
时间: 2024-10-14 12:09:55 浏览: 47
当你有一个圆,其半径为 \( r \),可以使用下面的公式来计算圆的周长 \( C \) 和面积 \( A \):
1. **周长** \( C = 2\pi r \)
2. **面积** \( A = \pi r^2 \)
其中 \( \pi \) 是圆周率,通常取值约为 3.14159。
例如,如果你的半径 \( r = 5 \),那么周长会是 \( 2 \times 3.14159 \times 5 \approx 31.42 \),面积则是 \( 3.14159 \times 5^2 \approx 78.54 \)。
为了保留两位小数,你可以使用四舍五入的方法,如 `math.floor()` 或 `round()` 函数(如果是在编程语言中)来处理结果。
如果你想直接看到计算过程,我可以展示一个简单的代码片段(假设用Python):
```python
import math
radius = float(input("请输入圆的半径:"))
circumference = 2 * math.pi * radius
area = math.pi * (radius ** 2)
# 保留两位小数
circumference_rounded = round(circumference, 2)
area_rounded = round(area, 2)
print(f"圆的周长是:{circumference_rounded}")
print(f"圆的面积是:{area_rounded}")
```
相关问题
长万形和园形都属于儿何图形,都有周长和面积,并日它们都有自己的周长和面积计算公式。使用抽缘 类的知识设计一个程序,可以计算不同图形的面积和周长
好的,我可以为您设计一个计算不同图形面积和周长的程序。首先,我们需要定义一个抽象类 Shape,它包含两个纯虚函数 getArea() 和 getPerimeter(),分别用于计算图形的面积和周长。然后,我们可以定义不同的子类,如 Rectangle、Circle、Triangle 等,它们都继承自 Shape 类,并实现自己的 getArea() 和 getPerimeter() 函数。
以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Shape {
public:
virtual double getArea() = 0;
virtual double getPerimeter() = 0;
};
class Rectangle : public Shape {
public:
Rectangle(double w, double h) : width(w), height(h) {}
double getArea() { return width * height; }
double getPerimeter() { return 2 * (width + height); }
private:
double width, height;
};
class Circle : public Shape {
public:
Circle(double r) : radius(r) {}
double getArea() { return M_PI * radius * radius; }
double getPerimeter() { return 2 * M_PI * radius; }
private:
double radius;
};
class Triangle : public Shape {
public:
Triangle(double a, double b, double c) : sideA(a), sideB(b), sideC(c) {}
double getArea() {
double p = (sideA + sideB + sideC) / 2;
return sqrt(p * (p - sideA) * (p - sideB) * (p - sideC));
}
double getPerimeter() { return sideA + sideB + sideC; }
private:
double sideA, sideB, sideC;
};
int main() {
Shape* shapes[3];
shapes[0] = new Rectangle(3, 4);
shapes[1] = new Circle(2);
shapes[2] = new Triangle(3, 4, 5);
for (int i = 0; i < 3; i++) {
cout << "Shape " << i+1 << " area: " << shapes[i]->getArea() << endl;
cout << "Shape " << i+1 << " perimeter: " << shapes[i]->getPerimeter() << endl;
delete shapes[i];
}
return 0;
}
```
这个程序定义了三个子类 Rectangle、Circle 和 Triangle,它们分别代表矩形、圆形和三角形。在 main 函数中,我们创建了一个 Shape 类型的数组,其中存放了三个不同的图形。然后,我们遍历这个数组,分别调用每个图形的 getArea() 和 getPerimeter() 函数,输出它们的面积和周长。
输出结果如下:
```
Shape 1 area: 12
Shape 1 perimeter: 14
Shape 2 area: 12.5664
Shape 2 perimeter: 12.5664
Shape 3 area: 6
Shape 3 perimeter: 12
```
可以看到,程序成功计算了三个不同图形的面积和周长。
用java写出长万形和园形都属于儿何图形,都有周长和面积,并日它们都有自己的周长和面积计算公式。使用抽缘 类的知识设计一个程序,可以计算不同图形的面积和周长
好的,我可以回答这个问题。对于长方形和圆形,它们的周长和面积计算公式分别为:
长方形周长:2 * (长 + 宽)
长方形面积:长 * 宽
圆形周长:2 * π * 半径
圆形面积:π * 半径的平方
以下是一个使用抽象类的程序示例,可以计算不同图形的面积和周长:
abstract class Shape {
public abstract double getPerimeter();
public abstract double getArea();
}
class Rectangle extends Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double getPerimeter() {
return 2 * (length + width);
}
public double getArea() {
return length * width;
}
}
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getPerimeter() {
return 2 * Math.PI * radius;
}
public double getArea() {
return Math.PI * Math.pow(radius, 2);
}
}
public class Main {
public static void main(String[] args) {
Shape rectangle = new Rectangle(5, 10);
Shape circle = new Circle(3);
System.out.println("长方形周长:" + rectangle.getPerimeter());
System.out.println("长方形面积:" + rectangle.getArea());
System.out.println("圆形周长:" + circle.getPerimeter());
System.out.println("圆形面积:" + circle.getArea());
}
}
阅读全文
相关推荐
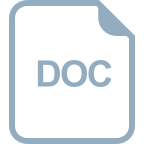
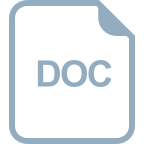
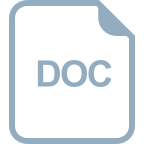
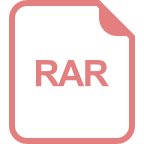
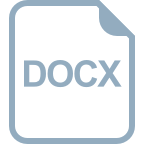
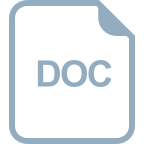
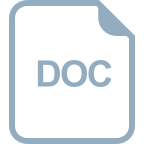
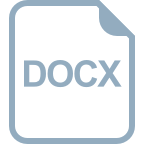
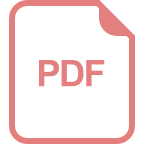
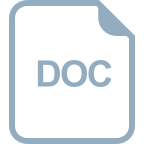
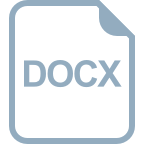
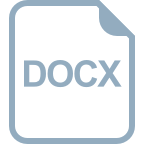
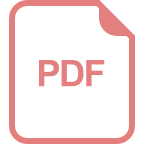
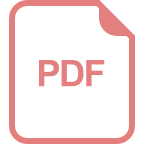
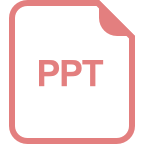