C# wpf中 鼠标停在按钮上 实现呼吸灯效果 离开后恢复 简单示例代码
时间: 2024-12-21 13:30:18 浏览: 14
在C# WPF中,要实现鼠标悬停在按钮上时显示呼吸灯效果(即闪烁效果),可以使用`MouseEnter`和`MouseLeave`事件以及一些动画操作。下面是一个简单的示例代码:
```csharp
<Window x:Class="BreathLightExample.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="450" Width="800">
<Button Name="myButton"
Content="点击我"
Background="{DynamicResource BreathLight}"
BorderBrush="Transparent"
MouseEnter="MyButton_MouseEnter"
MouseLeave="MyButton_MouseLeave">
</Button>
</Window>
// XAML头部添加动态资源
<Style TargetType="Button">
<Style.Triggers>
<Trigger Property="IsMouseOver" Value="True">
<Animation Duration="0:0:0.5" Storyboard="{StaticResource FlashStoryboard}" />
</Trigger>
</Style.Triggers>
</Style>
<!-- 在XAML.cs文件中编写事件处理和动画定义 -->
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
// 创建Storyboard实例
var flashStoryboard = new Storyboard();
var colorAnimation = new ColorAnimation
{
From = Colors.Transparent,
To = Colors.LightBlue,
RepeatBehavior = RepeatBehavior.Forever,
Duration = TimeSpan.FromSeconds(0.5),
};
// 添加动画到Storyboard
var colorAnimationTrack = new ColorAnimationUsingKeyFrames
{
KeyFrames =
{
new SolidColorBrushColorKeyFrame((double)0, Colors.Transparent),
new SolidColorBrushColorKeyFrame((double)0.5, Colors.LightBlue)
}
};
Storyboard.SetTargetProperty(colorAnimationTrack, new PropertyPath(Button.BackgroundProperty));
flashStoryboard.Children.Add(colorAnimationTrack);
flashStoryboard.Begin(this.myButton);
}
private void MyButton_MouseEnter(object sender, MouseEventArgs e)
{
// 当鼠标进入按钮,开始动画
((Storyboard)this.FindResource("FlashStoryboard")).Begin();
}
private void MyButton_MouseLeave(object sender, MouseEventArgs e)
{
// 当鼠标离开按钮,停止动画
((Storyboard)this.FindResource("FlashStoryboard")).Stop();
}
}
```
在这个例子中,当鼠标进入按钮区域时,会触发颜色动画,使背景色从透明变为浅蓝色,然后循环闪烁。鼠标移开时,动画会停止。注意这只是一个基础示例,你可以根据需要调整动画的颜色、持续时间和其他属性。
阅读全文
相关推荐






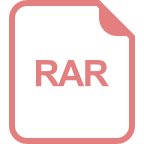




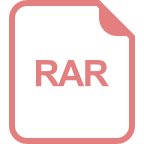

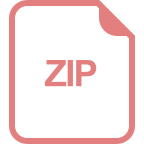


