第3题:编写一个MR程序将任务2操作的Hbase数据库中ORDER_BILL_RECODER表的数据,复制到一个新的表NEW_ORDER_BILL_RECODER中
时间: 2024-12-02 15:17:00 浏览: 17
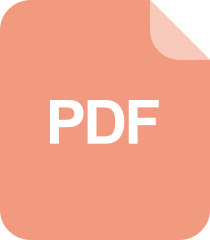
HBase:一个可扩展的、高性能分布式数据库
在Hadoop MapReduce框架下,编写一个程序来复制数据通常涉及到两个主要阶段:Map和Reduce。以下是基本步骤:
1. **Map阶段**:
- 输入:从ORDER_BILL_RECODER表读取行键(row key)作为输入键(key),订单记录(order data)作为输入值(value)。
- Map函数:对于每个订单记录,Mapper会处理该记录并生成新的键值对。这通常是通过对记录做一些简单操作,比如提取字段,然后组合成新表所需的键(例如,旧表的订单ID可以作为新表的键),旧记录本身作为值。
```java
// 示例Mapper代码
public class OrderBillRecordMapper extends Mapper<LongWritable, Text, Text, Text> {
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String orderData = value.toString();
// 解析orderData,提取需要的字段
String newKey = ...; // 新表的键
context.write(new Text(newKey), Text.of(orderData));
}
}
```
2. **Shuffle and Reduce阶段**:
- Shuffle: 已经映射过的键值对会被排序并分发给Reducer。在这个阶段,所有具有相同键的原始键值对都会合并在一起。
- Reduce函数:接收的是相同的键和一组对应的值(这里是原始ORDER_BILL_RECODER表的记录)。Reduce负责整合这些值,生成最终的新条目并写入NEW_ORDER_BILL_RECODER表。
```java
// 示例Reducer代码
public class OrderBillRecordReducer extends Reducer<Text, Text, Text, Text> {
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
StringBuilder newRow = new StringBuilder();
for (Text value : values) {
newRow.append(value.toString());
}
context.write(key, newRow.toString());
}
}
```
3. **Job Configuration**:
- 配置InputFormat和OutputFormat,指定ORDER_BILL_RECODER表为输入源,NEW_ORDER_BILL_RECODER表为输出目标。
- 启动Job,并运行整个MapReduce作业。
```java
Configuration conf = new Configuration();
conf.set("mapreduce.input.format.class", "org.apache.hadoop.hbase.mapreduce.TableInputFormat");
conf.set("mapreduce.output.format.class", "org.apache.hadoop.hbase.mapreduce.TableOutputFormat");
...
Job job = Job.getInstance(conf, "Copy ORDER_BILL_RECODER to NEW_ORDER_BILL_RECODER");
job.setJarByClass(MyJob.class);
InputTable inputTable = new HTable(conf, "ORDER_BILL_RECODER");
OutputTable outputTable = new HTable(conf, "NEW_ORDER_BILL_RECODER");
...
job.waitForCompletion(true);
```
阅读全文
相关推荐
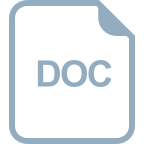
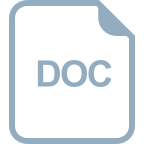
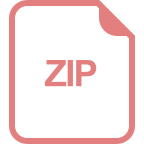
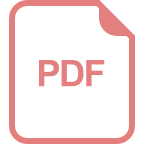
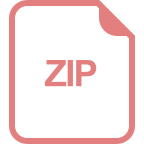
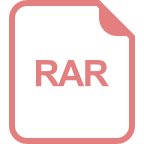
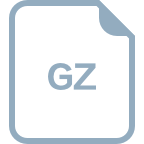
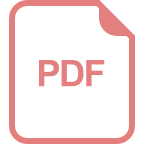
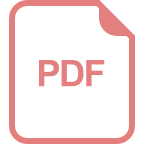
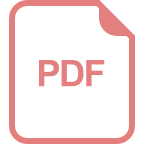
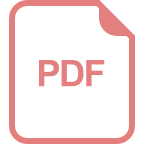
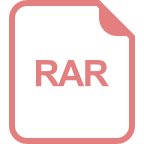
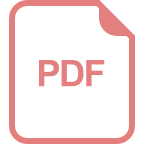
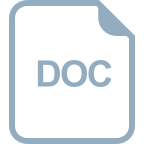
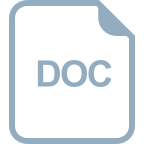