yolov8如何实现人脸检测
时间: 2024-03-02 07:46:58 浏览: 33
YOLOv8是一种用于目标检测的深度学习模型,可以用于人脸检测。下面是使用YOLOv8实现人脸检测的步骤[^1][^2]:
1. 下载YOLOv8的代码和预训练权重。可以从GitHub上的YOLOv8仓库下载代码,并从YOLO官方网站下载预训练权重。
2. 安装依赖项。在运行YOLOv8之前,需要安装一些依赖项,例如OpenCV、NumPy等。
3. 加载模型和权重。使用代码加载YOLOv8模型和预训练权重。
4. 读取图像。将待检测的图像加载到内存中。
5. 图像预处理。对图像进行预处理,例如缩放、归一化等。
6. 运行模型。将预处理后的图像输入到YOLOv8模型中,运行模型并获取检测结果。
7. 解析结果。解析模型输出的结果,提取人脸检测框的位置和置信度。
8. 绘制检测结果。将检测结果绘制在原始图像上,可以使用OpenCV等库进行绘制。
9. 显示结果。将绘制好的图像显示出来,可以保存结果或实时展示。
下面是一个使用YOLOv8实现人脸检测的Python代码示例:
```python
import cv2
import numpy as np
# 加载模型和权重
net = cv2.dnn.readNetFromDarknet('yolov3.cfg', 'yolov3.weights')
# 读取图像
image = cv2.imread('image.jpg')
# 图像预处理
blob = cv2.dnn.blobFromImage(image, 1/255, (416, 416), swapRB=True, crop=False)
# 运行模型
net.setInput(blob)
output_layers_names = net.getUnconnectedOutLayersNames()
layer_outputs = net.forward(output_layers_names)
# 解析结果
boxes = []
confidences = []
class_ids = []
for output in layer_outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * image.shape[1])
center_y = int(detection[1] * image.shape[0])
width = int(detection[2] * image.shape[1])
height = int(detection[3] * image.shape[0])
left = int(center_x - width / 2)
top = int(center_y - height / 2)
boxes.append([left, top, width, height])
confidences.append(float(confidence))
class_ids.append(class_id)
# 非最大抑制
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 绘制检测结果
font = cv2.FONT_HERSHEY_PLAIN
colors = np.random.uniform(0, 255, size=(len(boxes), 3))
for i in range(len(boxes)):
if i in indexes:
x, y, w, h = boxes[i]
label = str(class_ids[i])
confidence = confidences[i]
color = colors[i]
cv2.rectangle(image, (x, y), (x + w, y + h), color, 2)
cv2.putText(image, label, (x, y - 5), font, 1, color, 1)
# 显示结果
cv2.imshow("Image", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
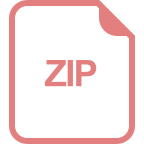
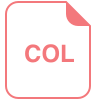
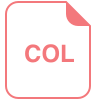
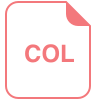
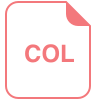
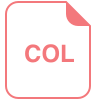









