python程序设计,请使用函数计算圆的面积和长方形面积。
时间: 2024-10-13 08:17:14 浏览: 21
在Python程序设计中,我们可以定义两个函数分别用于计算圆形和长方形的面积。这里是一个简单的例子:
```python
import math
# 定义计算圆面积的函数
def circle_area(radius):
return math.pi * (radius ** 2)
# 定义计算长方形面积的函数
def rectangle_area(length, width):
return length * width
# 示例用法
circle_radius = 5
print("圆的面积:", circle_area(circle_radius))
rectangle_length = 10
rectangle_width = 8
print("长方形的面积:", rectangle_area(rectangle_length, rectangle_width))
```
在这个例子中,`circle_area()`函数接收半径作为参数,并返回计算出的圆的面积;而`rectangle_area()`函数则接受长和宽作为参数,返回长方形的面积。你可以根据需要调用这两个函数,并传入相应的尺寸。
相关问题
python程序设计。请使用函数计算圆的面积和长方形面积。
Python是一种高级、动态类型的解释型编程语言,以其简洁易读的语法和强大的库支持而著名。在Python中,我们可以很容易地编写函数来计算几何形状如圆形和长方形的面积。
首先,我们创建一个函数`circle_area()`来计算圆的面积,公式是πr²,其中r是半径。假设π的近似值可以设置为3.14159:
```python
import math
def circle_area(radius):
area = math.pi * radius ** 2
return area
```
然后,我们创建另一个函数`rectangle_area()`来计算长方形的面积,它需要两个参数:长度`length`和宽度`width`:
```python
def rectangle_area(length, width):
area = length * width
return area
```
你可以像下面这样调用这两个函数:
```python
radius = float(input("请输入圆的半径: "))
area_circle = circle_area(radius)
print(f"圆的面积是: {area_circle}")
length = float(input("请输入长方形的长度: "))
width = float(input("请输入长方形的宽度: "))
area_rectangle = rectangle_area(length, width)
print(f"长方形的面积是: {area_rectangle}")
```
面积计算:能够计算出 三角形,圆形,长方形,梯形等形状的 面积,要求用户输入图形类型(选择),并输入图形的关键参数。 体积计算:能够计算出 长方体,圆柱体,球体,圆锥等立体的体积,要求用户输入立体类型(选择),并输入关键参数。写一个python程序,使用图形设计界面
好的,以下是一个使用图形界面的 Python 程序实现面积计算和体积计算功能,具体注释见代码中:
```python
import tkinter as tk
import math
# 计算三角形面积
def triangle_area():
a = float(a_entry.get())
h = float(h_entry.get())
area = 0.5 * a * h
result_label.config(text="三角形的面积为:%.2f" % area)
# 计算圆形面积
def circle_area():
r = float(r_entry.get())
area = math.pi * r * r
result_label.config(text="圆的面积为:%.2f" % area)
# 计算长方形面积
def rectangle_area():
l = float(l_entry.get())
w = float(w_entry.get())
area = l * w
result_label.config(text="长方形的面积为:%.2f" % area)
# 计算梯形面积
def trapezoid_area():
a = float(a_entry.get())
b = float(b_entry.get())
h = float(h_entry.get())
area = 0.5 * (a + b) * h
result_label.config(text="梯形的面积为:%.2f" % area)
# 计算长方体体积
def cuboid_volume():
l = float(l_entry.get())
w = float(w_entry.get())
h = float(h_entry.get())
volume = l * w * h
result_label.config(text="长方体的体积为:%.2f" % volume)
# 计算圆柱体体积
def cylinder_volume():
r = float(r_entry.get())
h = float(h_entry.get())
volume = math.pi * r * r * h
result_label.config(text="圆柱体的体积为:%.2f" % volume)
# 计算球体体积
def sphere_volume():
r = float(r_entry.get())
volume = 4 / 3 * math.pi * r * r * r
result_label.config(text="球体的体积为:%.2f" % volume)
# 计算圆锥体积
def cone_volume():
r = float(r_entry.get())
h = float(h_entry.get())
volume = 1 / 3 * math.pi * r * r * h
result_label.config(text="圆锥的体积为:%.2f" % volume)
# 创建窗口
root = tk.Tk()
root.title("面积和体积计算器")
# 创建控件
type_label = tk.Label(root, text="请选择图形类型:")
type_label.grid(row=0, column=0, padx=10, pady=5)
type_var = tk.StringVar()
type_var.set("triangle")
type_menu = tk.OptionMenu(root, type_var, "triangle", "circle", "rectangle", "trapezoid", "cuboid", "cylinder", "sphere", "cone")
type_menu.grid(row=0, column=1, padx=10, pady=5)
a_label = tk.Label(root, text="底边长:")
a_entry = tk.Entry(root)
h_label = tk.Label(root, text="高:")
h_entry = tk.Entry(root)
r_label = tk.Label(root, text="半径:")
r_entry = tk.Entry(root)
l_label = tk.Label(root, text="长度:")
l_entry = tk.Entry(root)
w_label = tk.Label(root, text="宽度:")
w_entry = tk.Entry(root)
b_label = tk.Label(root, text="上底长:")
b_entry = tk.Entry(root)
a_label.grid(row=1, column=0, padx=10, pady=5)
a_entry.grid(row=1, column=1, padx=10, pady=5)
h_label.grid(row=2, column=0, padx=10, pady=5)
h_entry.grid(row=2, column=1, padx=10, pady=5)
r_label.grid(row=1, column=0, padx=10, pady=5)
r_entry.grid(row=1, column=1, padx=10, pady=5)
l_label.grid(row=1, column=0, padx=10, pady=5)
l_entry.grid(row=1, column=1, padx=10, pady=5)
w_label.grid(row=2, column=0, padx=10, pady=5)
w_entry.grid(row=2, column=1, padx=10, pady=5)
b_label.grid(row=1, column=0, padx=10, pady=5)
b_entry.grid(row=1, column=1, padx=10, pady=5)
calculate_btn = tk.Button(root, text="计算", command=lambda: globals()[type_var.get() + "_area" if type_var.get() in ["triangle", "circle", "rectangle", "trapezoid"] else type_var.get() + "_volume"]())
calculate_btn.grid(row=3, column=0, padx=10, pady=5)
result_label = tk.Label(root, text="")
result_label.grid(row=3, column=1, padx=10, pady=5)
# 运行窗口
root.mainloop()
```
这个程序使用了 Tkinter 模块创建了一个简单的图形界面,用户可以通过下拉菜单选择要计算的图形类型,然后在相应的文本框中输入关键参数,点击“计算”按钮即可计算出面积或体积,结果会显示在标签中。注意,为了在程序中动态调用计算函数,我使用了一个字典和一个字符串拼接的方式来实现,这可能有一些风险,实际应用中需要更加谨慎。
阅读全文
相关推荐
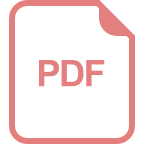
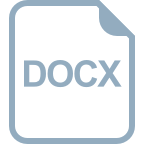
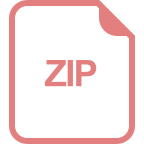
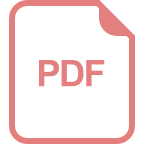
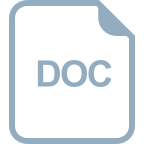
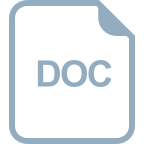










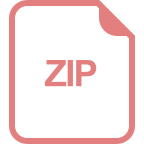