基于python,使用Beautiful Soup 4获取下厨房本周最受欢迎菜单,菜名,食材,链接,作者,使用Xpath获取下厨房本周最受欢迎菜单,菜名,食材,链接,作者使用正则表达式获取下厨房本周最受欢迎菜单,菜名,食材,链接,作者。请给出具体代码
时间: 2024-11-09 08:20:29 浏览: 12
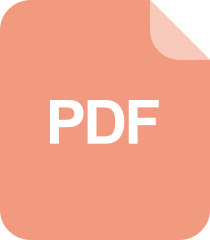
Python 使用Beautiful Soup 爬虫教程.pdf
首先,需要明确的是,下厨房网站的数据结构可能会变化,这可能导致直接使用XPath或正则表达式无法直接获取到所需信息。不过,我会为你展示如何使用BeautifulSoup库从下厨房抓取数据的基本步骤。由于实际URL和HTML结构未提供,我将假设一个示例URL和HTML结构。
对于基于BeautifulSoup的获取:
```python
import requests
from bs4 import BeautifulSoup
# 假设这是下厨房的页面URL
url = "https://www.xiachufang.com/recipe/top_recipes"
# 发送GET请求
response = requests.get(url)
html_content = response.text
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(html_content, 'html.parser')
# 查找特定元素,如菜名、食材等,可能需要根据实际网页结构调整
recipe_cards = soup.find_all('div', class_='recipe-card') # 假设是这个类名
for card in recipe_cards:
title = card.find('h2', class_='title')['data-title'] # 获取菜名,通常在标题标签内有属性"data-title"
ingredients = card.find('span', class_='ingredients') # 食材在span标签内,假设类名为"ingredients"
link = card.find('a')['href'] # 链接在<a>标签内的href属性
author = card.find('p', class_='author')['data-username'] # 作者可能在p标签内,有"data-username"属性
print(f"菜名:{title}")
print(f"食材:{ingredients.strip()}")
print(f"链接:{link}")
print(f"作者:{author}\n")
```
对于使用XPath:
```python
import requests
from lxml import etree
from urllib.parse import urljoin
# ... (同上) ...
def get_xpath_data(response):
html_doc = etree.HTML(response.text)
recipes = html_doc.xpath('//div[@class="recipe-card"]')
for recipe in recipes:
title = recipe.xpath('./h2[@class="title"]/@data-title')[0]
ingredients = recipe.xpath('./span[@class="ingredients"]/text()')[0].strip()
link = urljoin(url, recipe.xpath('./a/@href')[0])
author = recipe.xpath('./p[@class="author"]/@data-username')[0]
yield {
"title": title,
"ingredients": ingredients,
"link": link,
"author": author
}
# ... (同上) ...
for data in get_xpath_data(response):
# 打印数据
print(data)
```
对于使用正则表达式,由于HTML的复杂性和不确定性,这种方法通常不是最佳选择,但如果确实能找到稳定的匹配模式,可以尝试:
```python
import re
import requests
# ... (同上) ...
pattern_title = r'data-title="(.*?)"'
pattern_ingredients = r'<span class="ingredients">(.*?)</span>'
pattern_author = r'data-username="(.*?)"'
recipe_data = {}
response = requests.get(url)
content = response.text
titles = re.findall(pattern_title, content)
ingredients = re.findall(pattern_ingredients, content)
authors = re.findall(pattern_author, content)
i = 0
for _ in range(len(recipe_cards)):
recipe_data["title"] = titles[i]
recipe_data["ingredients"] = ingredients[i].strip()
recipe_data["link"] = "..." # 此处应根据实际情况替换,比如使用findall后的链接
recipe_data["author"] = authors[i]
print(recipe_data)
i += 1
```
请注意,上述代码中的某些部分需要根据实际下厨房网页结构调整。如果你能提供具体的HTML片段,我可以提供更精确的帮助。
阅读全文
相关推荐
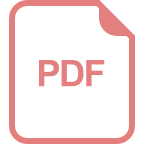
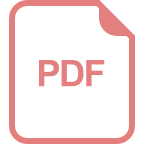
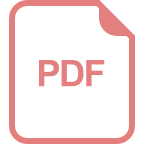
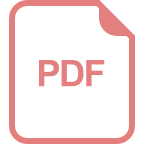
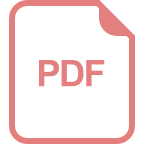
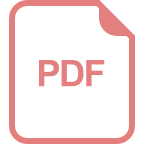
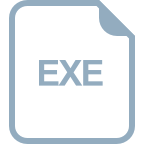
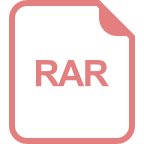
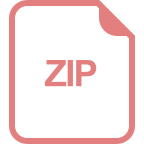
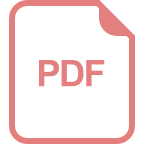
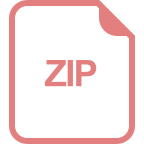