运用C语言,分别通过头差法,尾差法建立字符链表,要求至少a~e五个元素,通过头指针挨个输入元素并最终销毁。
时间: 2024-09-18 15:16:40 浏览: 53
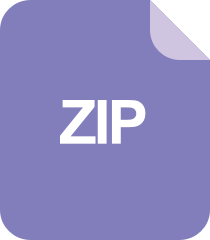
头插法、尾插法、链表长度test-c-master.zip
在C语言中,我们可以使用链表结构来创建字符链表。这里我们将演示两种方法:头插法(也叫首部插入法,即在头节点之后插入)和尾插法(即在最后一个节点之后插入),并且会处理循环结束后的链表销毁。
**一、头插法(头差法)**
1. 首先,定义链表节点结构:
```c
typedef struct ListNode {
char data;
struct ListNode* next;
} ListNode;
```
2. 创建链表头节点:
```c
ListNode* head = (ListNode*)malloc(sizeof(ListNode));
head->data = 'a';
head->next = NULL;
```
3. 循环读取输入并插入头节点:
```c
char input;
while ((input = getchar()) != '\n' && input != EOF) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = input;
newNode->next = head->next;
head->next = newNode;
}
```
4. 输入结束后,释放所有节点:
```c
ListNode* temp = head;
while (temp != NULL) {
ListNode* nextTemp = temp->next;
free(temp);
temp = nextTemp;
}
free(head);
```
**二、尾插法(尾差法)**
1. 初始化链表尾节点:
```c
ListNode* tail = head;
tail->next = NULL;
```
2. 输入循环:
```c
while ((input = getchar()) != '\n' && input != EOF) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = input;
newNode->next = tail->next;
tail->next = newNode;
tail = newNode;
}
```
3. 释放节点同上,这里不再赘述。
阅读全文
相关推荐
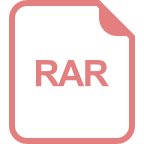
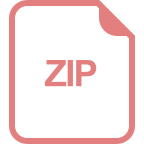
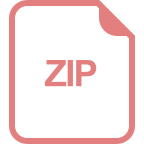
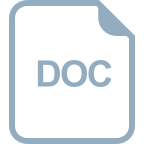
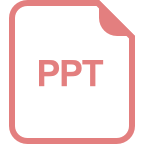
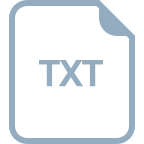
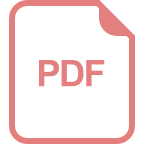
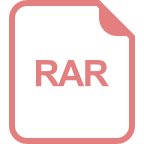
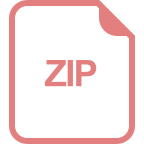
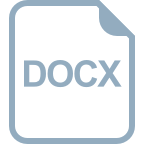
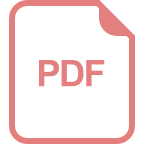
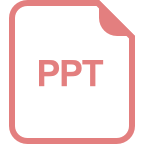
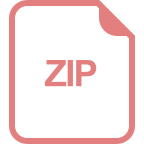
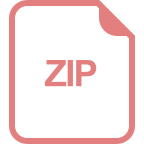