解释文件中第43页及之后的内容
时间: 2024-10-27 12:15:28 浏览: 9
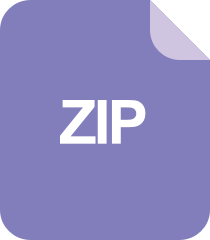
暴风43R4 配屏T430HVN01.272 机编60000AM3300 3302 屏参30165001 30174402物料号
### 文件《Chapter 7.pdf》第43页及之后的内容摘要:
#### 第43页:常量参数(Const Parameters)
- **目的**:防止函数内部修改传递进来的数组。
- **实现方式**:在函数声明和定义时,在数组参数前加上 `const` 关键字。
- **示例代码**:
```cpp
#include <iostream>
using namespace std;
void p(const int list[], int arraySize) {
// 尝试修改数组会导致编译错误
list[0] = 100; // 编译错误!
}
int main() {
int numbers[5] = {1, 4, 3, 6, 8};
p(numbers, 5);
return 0;
}
```
#### 第45页:从函数返回数组(Returning an Array from a Function)
- **限制**:C++ 不允许直接返回一个数组。
- **解决方案**:通过传递两个数组参数来实现数组的反转功能。
- **示例代码**:
```cpp
#include <iostream>
using namespace std;
// newList 是 list 的反转
void reverse(const int list[], int newList[], int size) {
for (int i = 0, j = size - 1; i < size; i++, j--) {
newList[j] = list[i];
}
}
void printArray(const int list[], int size) {
for (int i = 0; i < size; i++)
cout << list[i] << " ";
}
int main() {
const int SIZE = 6;
int list[] = {1, 2, 3, 4, 5, 6};
int newList[SIZE];
reverse(list, newList, SIZE);
cout << "The original array: ";
printArray(list, SIZE);
cout << endl;
cout << "The reversed array: ";
printArray(newList, SIZE);
cout << endl;
return 0;
}
```
#### 第48页:追踪反转函数(Trace the Reverse Function)
- **步骤**:
1. 初始化两个数组 `list` 和 `newList`。
2. 调用 `reverse` 函数,将 `list` 反转并存储到 `newList` 中。
3. 在 `reverse` 函数内部,使用两个索引 `i` 和 `j` 分别从头和尾遍历数组,逐个元素进行赋值。
- **示例追踪**:
- 初始状态:
```cpp
list: [1, 2, 3, 4, 5, 6]
newList:[0, 0, 0, 0, 0, 0]
```
- 迭代过程:
- `i = 0, j = 5`: `newList[5] = list[0]` → `[0, 0, 0, 0, 0, 1]`
- `i = 1, j = 4`: `newList[4] = list[1]` → `[0, 0, 0, 0, 2, 1]`
- `i = 2, j = 3`: `newList[3] = list[2]` → `[0, 0, 0, 3, 2, 1]`
- `i = 3, j = 2`: `newList[2] = list[3]` → `[0, 0, 4, 3, 2, 1]`
- `i = 4, j = 1`: `newList[1] = list[4]` → `[0, 5, 4, 3, 2, 1]`
- `i = 5, j = 0`: `newList[0] = list[5]` → `[6, 5, 4, 3, 2, 1]`
- 最终结果:
```cpp
list: [1, 2, 3, 4, 5, 6]
newList:[6, 5, 4, 3, 2, 1]
```
#### 第71页:搜索数组(Searching Arrays)
- **线性搜索(Linear Search)**:
- **原理**:顺序比较目标值与数组中的每个元素。
- **示例代码**:
```cpp
#include <iostream>
using namespace std;
int linearSearch(const int list[], int key, int arraySize) {
for (int i = 0; i < arraySize; i++) {
if (key == list[i])
return i;
}
return -1;
}
int main() {
int list[] = {4, 5, 1, 2, 9, -3};
cout << linearSearch(list, 2, 6) << endl;
return 0;
}
```
- **动画演示**:展示了线性搜索的过程,逐步比较目标值与数组中的每个元素。
#### 第76页:排序数组(Sorting Arrays)
- **选择排序(Selection Sort)**:
- **原理**:每次从未排序的部分找到最小元素,将其放到已排序部分的末尾。
- **示例代码**:
```cpp
#include <iostream>
using namespace std;
void selectionSort(double list[], int listSize) {
for (int i = 0; i < listSize - 1; i++) {
double currentMin = list[i];
int currentMinIndex = i;
for (int j = i + 1; j < listSize; j++) {
if (currentMin > list[j]) {
currentMin = list[j];
currentMinIndex = j;
}
}
if (currentMinIndex != i) {
list[currentMinIndex] = list[i];
list[i] = currentMin;
}
}
}
int main() {
double list[] = {2, 4.5, 5, 1, 2, -3.3};
selectionSort(list, 6);
for (int i = 0; i < 6; i++) {
cout << list[i] << " ";
}
cout << endl;
return 0;
}
```
#### 总结
- **数组操作**:包括声明、初始化、访问、常用操作(如求和、找最大值、随机填充等)、传递给函数、返回数组、搜索和排序。
- **注意事项**:C++ 不检查数组边界,使用 `const` 参数防止函数内部修改数组,以及如何通过传递多个数组参数来实现数组的返回。
阅读全文
相关推荐
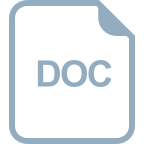
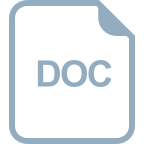
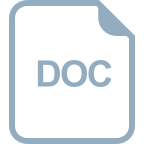
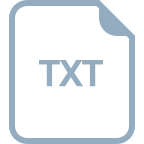
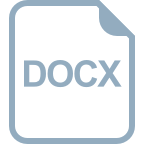
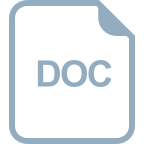
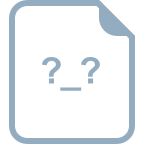
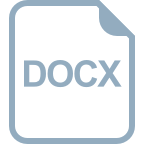
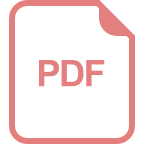
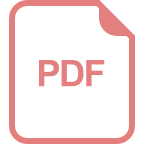
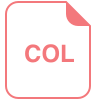




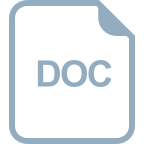
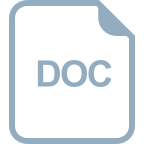