openfeign的使用示例及版本
时间: 2024-10-11 20:13:21 浏览: 9
OpenFeign是一个由Netflix开发的轻量级HTTP客户端库,它用于简化分布式系统中的服务发现和API调用。OpenFeign的核心思想是将RESTful API的调用抽象为Java方法的调用,通过注解驱动的方式生成服务契约。
以下是使用OpenFeign的基本步骤和一个简单的示例:
1. 添加依赖:在Maven项目中,添加`io.github.openfeign:openfeign-core`和`io.github.openfeign:openfeign-jackson`等相关的依赖。
```xml
<dependency>
<groupId>io.github.openfeign</groupId>
<artifactId>openfeign-core</artifactId>
<version>9.7.0</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.6</version>
</dependency>
```
2. 创建接口并使用@FeignClient注解声明远程服务:
```java
import feign.Feign;
import feign.Headers;
@FeignClient(name = "api-service", url = "http://example.com/api")
public interface ApiService {
@Headers("Content-Type: application/json")
@GetMapping("/users")
List<User> getUsers();
@PostMapping("/users")
User createUser(@RequestBody User user);
}
```
这里,我们创建了一个名为`ApiService`的接口,模拟了GET用户列表和POST新增用户的两个API。
3. 使用Feign实例化客户端并调用服务:
```java
import feign.Client;
import feign.Request;
import feign.Response;
import static java.util.Arrays.asList;
public class FeignExample {
private final Client client = new OkHttp();
public void callApi() {
ApiService apiService = Feign.builder()
.client(client)
.target(ApiService.class, "http://localhost:8080/api");
// 调用GET方法
Response response = apiService.getUsers().execute();
if (response.status() == 200) {
List<User> users = response.body();
System.out.println("Users: " + users);
}
// 调用POST方法
User newUser = ...; // 用户对象
response = apiService.createUser(newUser).execute();
System.out.println("Response status for POST: " + response.status());
}
}
```
在这个例子中,`callApi()`方法会根据接口定义去实际调用`api-service`服务。
阅读全文
相关推荐
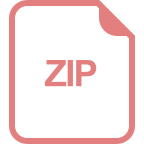
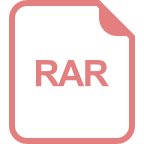
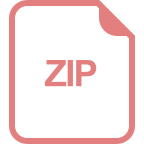






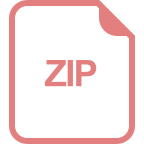
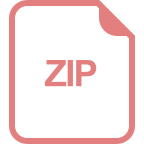






