用c语言编写函数,将一个顺序表 A(有n个元素且任何元素均不为 0),分拆成两个顺序表B和C。使A中大于0的元素存放在B中,小于0的元素存放在 C 中,返回顺序表B和C。
时间: 2024-10-10 19:06:03 浏览: 35
在C语言中,你可以通过遍历顺序表A并根据元素的正负值将它们分别添加到新的列表B和C中来实现这个功能。这里是一个简单的示例,假设我们已经有了顺序表结构体`Node`和两个空的顺序表`List B`和`List C`:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建顺序表的新节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 合并两个顺序表
void mergeLists(Node** listB, Node** listC) {
// 如果B为空,直接将C的内容添加到结果中
if (*listB == NULL && *listC != NULL) {
*listB = *listC;
} else { // 否则比较A、B、C的头节点,选择较小的一个添加到结果中
if (*listB == NULL || (*listC != NULL && (*listC)->data < (*listB)->data)) {
*listB = *listC;
}
while (*listB != NULL) {
Node* temp = *listB;
*listB = (*listB)->next; // 移动指针
Node* newNode = createNode(temp->data); // 创建新节点
newNode->next = *listC; // 将新节点添加到C的末尾
*listC = newNode;
}
}
}
// 分割顺序表A,大于0的元素放入B,小于0的元素放入C
void splitList(Node* A, Node** listB, Node** listC) {
Node* current = A;
while (current != NULL) {
if (current->data > 0) {
mergeLists(listB, listC);
*listB = createNode(current->data);
} else {
*listC = createNode(current->data);
}
current = current->next;
}
}
// 打印顺序表
void printList(Node* list) {
while (list != NULL) {
printf("%d -> ", list->data);
list = list->next;
}
printf("NULL\n");
}
int main() {
// 初始化A、B和C,这里省略实际数据填充部分
Node* A = ...;
Node* listB = NULL;
Node* listC = NULL;
splitList(A, &listB, &listC);
printList(listB);
printList(listC);
return 0;
}
```
在这个程序中,`splitList`函数会遍历输入顺序表A,并根据元素的正负值动态创建新的顺序表B和C。注意,这个例子假设了内存管理已妥善处理,实际应用中需要考虑内存分配和释放。
阅读全文
相关推荐
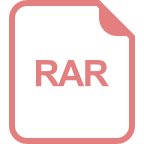





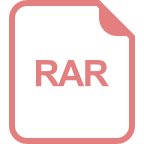
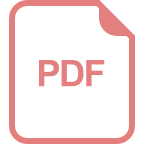

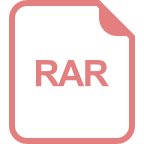
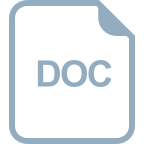

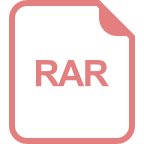
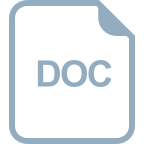





