用c语言创建一个升序排列、结点数据元素类型为整型的链表,并将数据元素输出
时间: 2024-09-25 16:14:18 浏览: 16
在 C 语言中,我们可以使用结构体定义节点并创建一个简单的单向递增链表。首先,我们需要定义一个结构体 `ListNode` 来表示链表的节点,包含一个整数值 `data` 和指向下一个节点的指针 `next`:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int data; // 节点值
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
// 创建新节点的函数
ListNode* createNode(int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (!newNode) {
printf("内存分配失败\n");
return NULL;
}
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 插入节点到链表的函数
void insertSortedToList(ListNode** head, int value) {
ListNode* current = *head;
if (!current || value <= current->data) {
*head = createNode(value);
return;
}
while (current->next && value > current->next->data) {
current = current->next;
}
current->next = createNode(value);
}
// 打印链表中的所有节点
void printList(ListNode* head) {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
int main() {
// 初始化空链表
ListNode* listHead = NULL;
// 插入一些数字并排序
insertSortedToList(&listHead, 5); // [5]
insertSortedToList(&listHead, 2); // [2, 5]
insertSortedToList(&listHead, 8); // [2, 5, 8]
// 打印排序后的链表
printList(listHead);
return 0;
}
```
在这个示例中,我们首先创建了一个空链表,然后插入了一些整数并保持它们按升序排列。`insertSortedToList` 函数负责找到合适的位置插入新节点,最后使用 `printList` 函数打印整个链表。
相关推荐
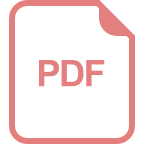
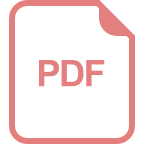
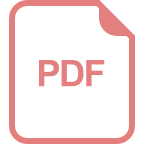














