Java8 获取当前时间前两个小时的时间 Date类型
时间: 2024-12-08 14:18:38 浏览: 14
在Java 8中,你可以使用`java.time`包下的`LocalDateTime`类和`Duration`类来获取当前时间前两个小时的时间。以下是具体的代码示例:
```java
import java.time.LocalDateTime;
import java.time.Duration;
import java.time.ZoneId;
public class Main {
public static void main(String[] args) {
// 获取当前时间
LocalDateTime now = LocalDateTime.now(ZoneId.systemDefault());
// 计算两个小时的时间差
Duration twoHours = Duration.ofHours(-2);
// 减去两小时并得到新的日期时间
LocalDateTime twoHoursAgo = now.minus(twoHours);
// 打印结果
System.out.println("Two hours ago: " + twoHoursAgo);
}
}
```
这段代码首先获取了系统默认时区下的当前时间,然后创建了一个表示负两个小时的`Duration`对象,最后从当前时间减去这个时间段,得到了两个小时前的时间。
相关问题
Java 获取当前时间前两个小时的时间 Date类型
在Java中获取当前时间前两个小时的时间,你可以使用`java.time`包下的`LocalDateTime`类结合`Duration`类来实现。以下是一个示例:
```java
import java.time.LocalDateTime;
import java.time.Duration;
import java.time.ZoneId;
public class Main {
public static void main(String[] args) {
// 获取当前日期和时间
LocalDateTime now = LocalDateTime.now();
// 减去两小时
Duration twoHours = Duration.ofHours(2);
LocalDateTime twoHoursBefore = now.minus(twoHours);
// 如果需要特定时区的时间,可以指定ZoneId,例如UTC
ZoneId zoneId = ZoneId.systemDefault();
LocalDateTime utcTwoHoursBefore = twoHoursBefore.atZone(zoneId);
// 输出结果
System.out.println("当前时间前两个小时: " + utcTwoHoursBefore.format(DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss")));
}
}
```
在这个例子中,我们首先获取当前的`LocalDateTime`,然后减去两个小时的`Duration`,得到两小时前的时间。如果需要考虑时区,可以将本地时间转换到指定的时区。
java获取2个小时前的时间 date类型
在Java中,你可以使用`java.time`包下的类来处理日期和时间操作。获取两个小时之前的当前时间,可以按照以下步骤:
```java
import java.time.LocalDateTime;
import java.time.ZoneId;
// 获取当前时间
LocalDateTime now = LocalDateTime.now();
// 减去两小时
long twoHoursInMilliseconds = 2 * 60 * 60 * 1000; // 2小时等于7200秒,每秒1000毫秒
LocalDateTime twoHoursAgo = now.minusSeconds(twoHoursInMilliseconds);
// 如果需要考虑时区,添加以下行
ZoneId systemTimeZone = ZoneId.systemDefault(); // 获取系统默认时区
LocalDateTime withTimeZone = twoHoursAgo.atZone(systemTimeZone); // 转换到时区
System.out.println(withTimeZone);
```
阅读全文
相关推荐
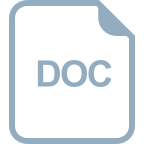
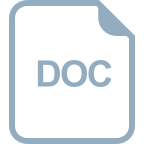
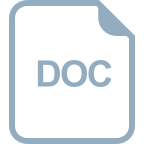













