设x和y为非负整数,对于集合{2*3*1 x≥0,y≥0},求集合中小于整数n的元素个数,并求这些元素从小到大排序的第m项元素用c++代码实现
时间: 2024-10-07 14:02:24 浏览: 27
在C++中,我们可以使用动态规划的思想来解决这个问题。首先我们需要计算小于n的所有可能的乘积组合,并存储在数组中。然后找到这个数组中第m小的元素。以下是代码的一个大致实现:
```cpp
#include <iostream>
#include <vector>
// Function to calculate the number of elements less than n in the given set
int countElements(int n) {
std::vector<int> dp(n+1, 0);
int product = 1;
for (int i = 1; i <= 3; ++i) {
// Multiply the current product by the next factor and update dp array
for (int j = product; j * i <= n; j += product) {
dp[j * i] += dp[j];
}
dp[product *= i]++;
}
return dp[n];
}
// Helper function to find the mth smallest element
int findMthElement(int m, const std::vector<int>& dp) {
std::vector<int> indices(dp.size(), -1); // Initialize indices with -1
indices[dp[0]] = 0;
for (int i = 1; i < dp.size(); ++i) {
if (indices[i - dp[i]] != -1) {
indices[i] = indices[i - dp[i]];
} else {
indices[i] = indices[i - 1] + 1;
}
}
if (m > dp.back()) {
return -1; // If m is larger than all possible products, return -1
}
return dp.begin() + indices[m]; // Return the index of the mth smallest element
}
int main() {
int n, m;
std::cout << "Enter a value for n: ";
std::cin >> n;
std::cout << "Enter a value for m: ";
std::cin >> m;
if (m <= 0 || n < 0) {
std::cout << "Invalid input! Both m and n must be non-negative integers.\n";
return 0;
}
int numElements = countElements(n);
if (m > numElements) {
std::cout << "There are not enough elements smaller than n (m=" << m << ").\n";
} else {
int mthElement = findMthElement(m, dp); // dp is the result from countElements
if (mthElement == -1) {
std::cout << "The mth element does not exist.\n";
} else {
std::cout << "The mth element is: " << mthElement << "\n";
}
}
return 0;
}
```
这段代码首先计算小于等于n的乘积的数量,然后通过构建一个索引辅助数据结构找到第m个小的元素。注意输入值需要满足题目条件,即n和m都是非负整数。
阅读全文
相关推荐
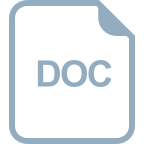
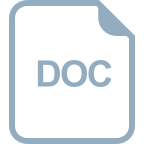
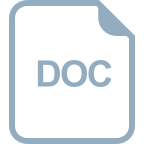



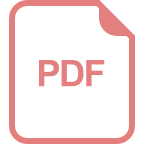
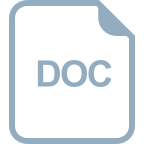
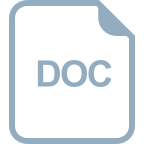
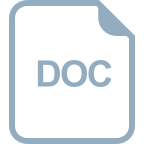
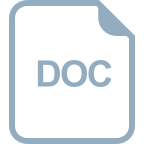
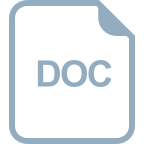
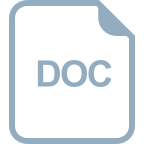
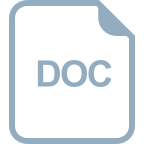
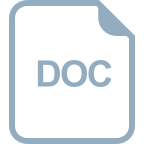
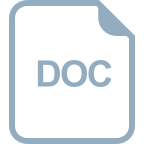
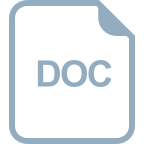
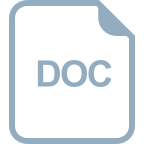
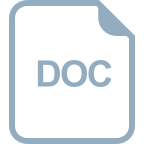