openssl命令aes加密
时间: 2024-09-02 18:03:49 浏览: 82
OpenSSL是一个强大的安全工具包,其中包括用于数据加密的命令行工具。AES(Advanced Encryption Standard),即高级加密标准,是一种对称密钥加密算法,常用于加密保护数据。
在命令行中使用openssl进行AES加密的基本步骤如下:
1. 加密文本:
```
openssl enc -aes-256-cbc -in plaintext.txt -aes-256-cbc` 指定了AES-256-CBC模式,这是一种常用的块密码模式。`-in plaintext.txt` 表示输入文件名,`-out ciphertext.bin` 是加密后的输出文件。`-pass pass:your_password` 设置了加密密码。
2. 解密:
```
openssl enc -d -aes-256-cbc -in ciphertext.bin -d` 参数表示解密操作。
注意:
- `your_password` 需要替换为你实际使用的密码。
- 对于安全性,建议使用环境变量而不是明文在命令行中指定密码。
相关问题
openssl进行AES加密
使用 OpenSSL 进行 AES 加密可以按照以下步骤进行:
1. 导入 OpenSSL 库:
```
#include <openssl/aes.h>
```
2. 定义 AES 加密的密钥和明文:
```
unsigned char *key = (unsigned char *)"01234567890123456789012345678901";
unsigned char *plaintext = (unsigned char *)"This is the plaintext.";
```
3. 定义 AES 加密的向量(IV):
```
unsigned char *iv = (unsigned char *)"0123456789012345";
```
4. 定义 AES 上下文结构体:
```
AES_KEY aes_key;
```
5. 初始化 AES 上下文:
```
AES_set_encrypt_key(key, 256, &aes_key);
```
6. 定义加密后的密文缓冲区:
```
unsigned char ciphertext[128];
```
7. 进行 AES 加密:
```
AES_cbc_encrypt(plaintext, ciphertext, strlen(plaintext), &aes_key, iv, AES_ENCRYPT);
```
完整的示例代码如下:
```
#include <openssl/aes.h>
#include <stdio.h>
#include <string.h>
int main()
{
unsigned char *key = (unsigned char *)"01234567890123456789012345678901";
unsigned char *plaintext = (unsigned char *)"This is the plaintext.";
unsigned char *iv = (unsigned char *)"0123456789012345";
AES_KEY aes_key;
AES_set_encrypt_key(key, 256, &aes_key);
unsigned char ciphertext[128];
AES_cbc_encrypt(plaintext, ciphertext, strlen(plaintext), &aes_key, iv, AES_ENCRYPT);
printf("Plaintext: %s\n", plaintext);
printf("Ciphertext: ");
for(int i = 0; i < strlen(plaintext); i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
return 0;
}
```
需要注意的是,上述示例代码中使用的是 AES-256 加密,如果需要使用其他加密长度,可以根据需要调整相关参数。同时,还需要注意密钥和向量的长度要符合 AES 加密标准。
openSSL实现AES加密
OpenSSL是一个开源的加密库,可以用来实现各种加密算法,包括AES加密。下面是一个使用OpenSSL实现AES加密的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <openssl/aes.h>
int main()
{
// 明文和密钥
const unsigned char plaintext[] = "Hello, world!";
const unsigned char key[] = "0123456789abcdef";
// 初始化AES加密上下文
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
// 加密
unsigned char ciphertext[16];
AES_encrypt(plaintext, ciphertext, &aes_key);
// 输出结果
printf("Plaintext: %s\n", plaintext);
printf("Ciphertext: ");
for (int i = 0; i < 16; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
return 0;
}
```
这段代码使用了OpenSSL提供的AES加密函数,其中`AES_set_encrypt_key()`用于初始化加密上下文,`AES_encrypt()`用于进行加密操作。需要注意的是,AES算法对明文的长度有要求,一般要求为16字节的倍数,所以在实际使用中需要进行填充。
阅读全文
相关推荐
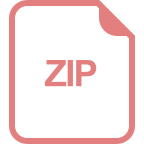
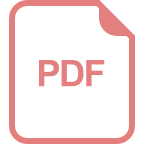
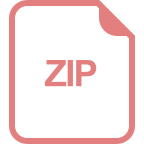
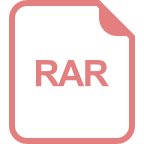
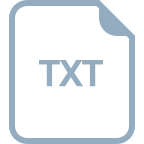
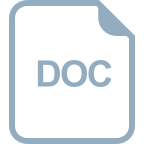




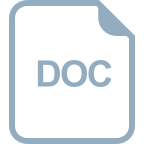
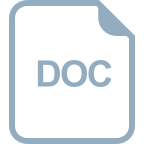
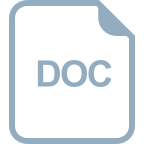
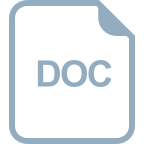
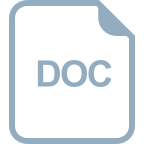

