RestTemplate发送https
时间: 2023-08-02 16:12:03 浏览: 93
您可以使用RestTemplate发送HTTPS请求。以下是一个示例:
```java
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.ResponseEntity;
import org.springframework.http.client.SimpleClientHttpRequestFactory;
import org.springframework.web.client.RestTemplate;
import javax.net.ssl.HttpsURLConnection;
import java.io.IOException;
import java.net.HttpURLConnection;
public class HttpsRequestExample {
public static void main(String[] args) {
// 创建RestTemplate实例
RestTemplate restTemplate = new RestTemplate();
// 创建HTTPS连接工厂
SimpleClientHttpRequestFactory requestFactory = new SimpleClientHttpRequestFactory();
requestFactory.setChunkSize(1024);
requestFactory.setConnectTimeout(5000); // 连接超时时间限制
requestFactory.setReadTimeout(5000); // 读取超时时间限制
// 配置HTTPS连接
try {
HttpsURLConnection.setDefaultHostnameVerifier((hostname, session) -> true);
requestFactory.setSSLSocketFactory(HttpsUtil.getSSLContext().getSocketFactory());
} catch (Exception e) {
e.printStackTrace();
}
restTemplate.setRequestFactory(requestFactory);
// 设置请求头
HttpHeaders headers = new HttpHeaders();
headers.set("Content-Type", "application/json");
// 发送GET请求
String url = "https://example.com/api/resource";
HttpEntity<String> entity = new HttpEntity<>(headers);
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, entity, String.class);
// 处理响应结果
if (response.getStatusCode() == HttpStatus.OK) {
String responseBody = response.getBody();
System.out.println(responseBody);
} else {
System.out.println("Request failed with status code: " + response.getStatusCode());
}
}
}
```
在上面的示例中,我们创建了一个RestTemplate实例,并通过SimpleClientHttpRequestFactory设置HTTPS连接。然后,我们设置请求头,使用exchange方法发送GET请求,并处理响应结果。
请注意,您需要导入相关的依赖项,例如Spring Web和Apache HttpClient。此外,还需要实现一个HttpsUtil类来获取SSLContext以配置HTTPS连接。这里只提供了一个简单的示例,您可能需要根据您的具体情况进行调整。
阅读全文
相关推荐













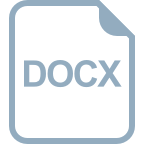