使用c#结合opencv实现车牌识别,给出能运行的代码
时间: 2024-09-29 16:09:26 浏览: 147
在C#中结合OpenCV进行车牌识别通常需要几个步骤:首先安装必要的库,然后加载图像,预处理图像以便于检测,接着使用OpenCV提供的特征检测算法(如HOG + SVM)或深度学习模型(比如YOLOv3或TensorFlow等)来进行车牌区域的定位,最后对定位到的区域进行字符分割和识别。
下面是一个简单的示例,展示如何使用C#通过Emgu CV库(OpenCV的.NET版本)进行车牌识别的基础框架。请注意,这只是一个简化的例子,实际的车牌识别项目会更复杂,并且需要训练好的模型文件:
```csharp
using Emgu.CV;
using Emgu.CV.CvEnum;
using Emgu.CV.Structure;
// ... (其他所需命名空间)
public void DetectLicensePlate(string imagePath)
{
// 读取图片
var image = new Image<Bgr, byte>(imagePath);
// 转换为灰度图并调整对比度增强边缘
var grayImage = image.Convert<Gray, byte>();
var adaptiveThresholdedImage = grayImage.AdaptiveThreshold(255, AdaptiveThresholdType.GaussianC, ThresholdType.Binary, 9, 3);
// 寻找可能的候选框 - 这里可以使用霍夫变换或者基于模板匹配的方法
var contourFinder = new SimpleBlobDetector();
var contours = contourFinder.Detect(adaptiveThresholdedImage);
// 遍历每个轮廓,检查是否满足车牌尺寸
foreach (var contour in contours)
{
Rectangle rect = ApproximationAccuracy.Simple.ContourToRectangle(contour);
if (IsCandidateRect(rect, image.Width)) // 自定义判断候选框是否合理
{
// 对选中的矩形区域进行进一步识别(例如,切割、 OCR)
// 这部分需要一个针对车牌字符的OCR引擎或已训练的模型
// ...
// 识别结果
string licensePlateText = RecognizeCharsFromRegion(image, rect); // 模拟函数,实际上这里会使用OCR
Console.WriteLine($"Detected License Plate: {licensePlateText}");
}
}
}
private bool IsCandidateRect(Rectangle rect, int maxWidth)
{
// 检查矩形的宽高比以及它在图片中的位置,确保是可能的车牌
double aspectRatio = rect.Width / rect.Height;
return rect.Width >= 80 && rect.Width <= maxWidth * 0.75 && aspectRatio > 1.6; // 示例条件,可根据实际情况调整
}
// 这里只是一个模拟,你需要替换为实际的字符识别方法
private string RecognizeCharsFromRegion(Image<Bgr, byte> img, Rectangle region)
{
// 使用OpenCV或其他OCR技术识别字符
// 这部分通常是基于机器学习或者深度学习的,不是简单的一维数组操作
throw new NotImplementedException();
}
```
阅读全文
相关推荐
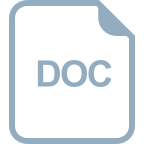
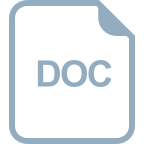
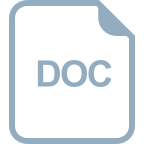
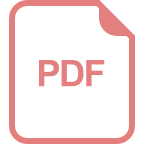
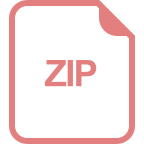
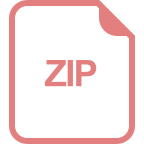
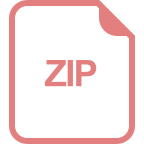
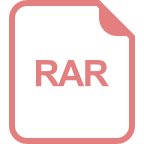
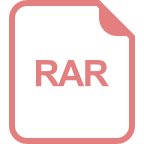
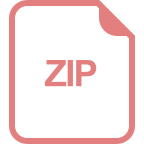
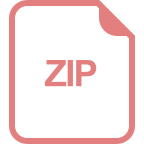
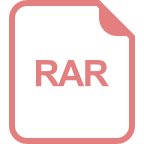
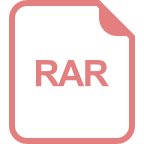
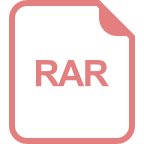
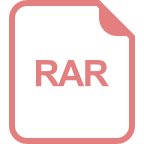
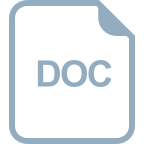
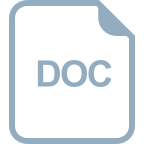
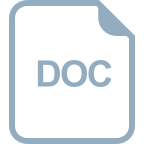
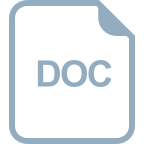