编写一个能读取灰度图像和彩色图像文件的程序,并在屏幕上进行显示。如果是彩色图像,则屏幕上除了显示彩色图像外,同时显示出三基色分量图像。
时间: 2024-09-22 16:04:07 浏览: 54
编写这样的程序需要使用一些图像处理库,比如Python的PIL(Pillow)或OpenCV。这里是一个简单的例子,我们将展示如何在Python中使用PIL来实现这个功能:
首先,确保已安装Pillow库,如果没有,可以使用pip install pillow命令安装。
```python
from PIL import Image
def display_image(file_path):
try:
# 打开图像文件
image = Image.open(file_path)
# 检查图像是否为RGB格式(彩色)
if image.mode == 'RGB':
# 显示原图
image.show()
# 分离色彩通道并分别显示
red_channel = image.convert('L') # L代表灰度模式
green_channel = image.convert('L')
blue_channel = image.convert('L')
red_channel.show(title='Red Channel')
green_channel.show(title='Green Channel')
blue_channel.show(title='Blue Channel')
# 如果是灰度图像,直接显示
elif image.mode == 'L':
image.show()
else:
print(f"Unsupported image mode: {image.mode}. Showing grayscale representation.")
image.show() # 将非RGB模式转换为灰度并显示
except Exception as e:
print(f"Error reading the file: {e}")
# 调用函数,传入你要显示的图片路径
file_path = input("Enter path to your image (gray or color): ")
display_image(file_path)
```
在这个示例中,用户会被提示输入图像文件路径,然后程序会尝试打开它。如果是彩色图像,它会显示原图以及三个单独的色彩通道;如果是灰度图像,则只显示原图。如果遇到无法处理的图像模式,程序会将图像转换为灰度并显示。
阅读全文
相关推荐
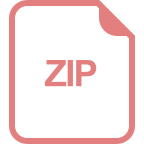
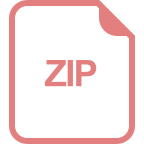
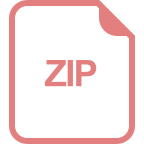



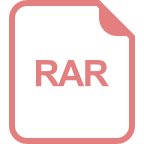











