用c++写一个输入运算符重载的例子
时间: 2024-09-24 17:19:15 浏览: 40
在C++中,你可以通过`operator=`运算符重载来处理输入流(如cin)。下面是一个简单的例子,演示如何重载`+=`操作符,使得我们可以从标准输入读取两个整数并相加:
```cpp
#include <iostream>
class Integer {
private:
int value;
public:
// 构造函数
Integer(int val = 0) : value(val) {}
// 输入运算符重载
friend std::istream& operator>>(std::istream& in, Integer& num) {
in >> num.value;
return in; // 返回流对象以便链式操作
}
// 自增运算符重载
Integer& operator+=(const Integer& other) {
value += other.value;
return *this;
}
// 输出结果
void print() const {
std::cout << "Integer: " << value << std::endl;
}
};
int main() {
Integer a, b;
std::cout << "Enter first number: ";
std::cin >> a; // 读取第一个数
std::cout << "Enter second number: ";
std::cin >> b; // 读取第二个数
// 使用自增运算符将两个数相加
a += b;
// 打印结果
a.print();
return 0;
}
```
在这个例子中,我们首先定义了输入运算符`>>`,当它用于`Integer`对象时,会从`in`流中读取值到`value`属性。然后,我们重载了`+=`运算符,使其可以链式地将两个`Integer`对象相加。
阅读全文
相关推荐
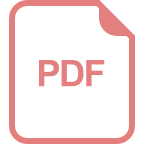
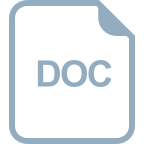
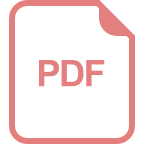






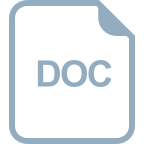
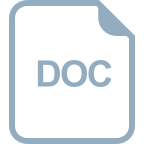
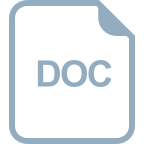
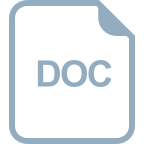
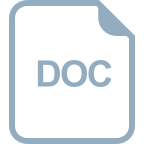
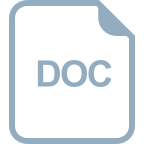
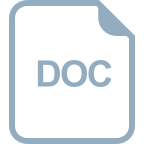
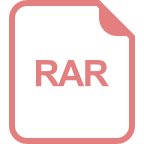
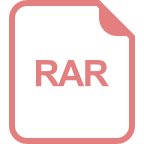