如何用c语言在单链表中实现元素的插入和删除操作?
时间: 2024-10-09 08:00:43 浏览: 6
在C语言中,我们可以使用结构体定义链表节点,然后为链表提供插入(insert)和删除(delete)元素的方法。这里我会给你一个基本的示例,包括头结点的定义、链表的创建以及插入和删除节点的操作。
首先,我们定义一个链表节点结构:
```c
struct Node {
int data; // 数据域
struct Node* next; // 指针域,指向下一个节点
};
```
接下来,我们创建一个函数来初始化一个空链表:
```c
// 创建一个空链表
struct Node* createList() {
return NULL;
}
```
用于插入新元素的函数:
```c
// 插入元素到链表末尾
void insertNode(struct Node** head, int data) {
if (*head == NULL) {
*head = (struct Node*)malloc(sizeof(struct Node));
(*head)->data = data;
(*head)->next = NULL;
} else {
struct Node* temp = *head;
while (temp->next != NULL)
temp = temp->next;
temp->next = (struct Node*)malloc(sizeof(struct Node));
temp->next->data = data;
temp->next->next = NULL;
}
}
```
删除特定元素的函数(假设我们要删除的数据已存在):
```c
// 删除链表中的指定元素
void deleteNode(struct Node** head, int key) {
if (*head == NULL)
return;
if ((*head)->data == key) {
struct Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
struct Node* prev = *head;
struct Node* curr = (*head)->next;
while (curr != NULL && curr->data != key) {
prev = curr;
curr = curr->next;
}
if (curr == NULL)
return;
prev->next = curr->next;
free(curr);
}
```
现在你可以使用这些函数来创建、插入和删除元素了。记得在使用完后释放内存,避免内存泄漏。
相关推荐
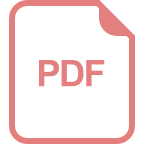
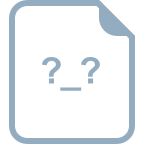
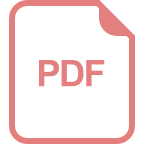
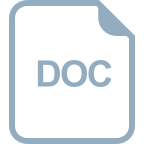
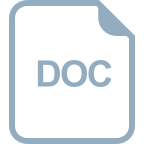
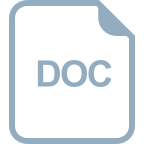
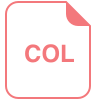







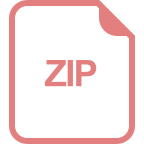
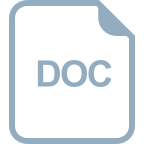
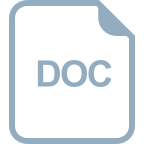
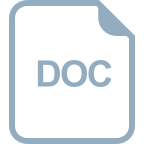
