android的fragement+4个button实现点击按钮背景颜色发生改变
时间: 2024-09-15 19:04:05 浏览: 44
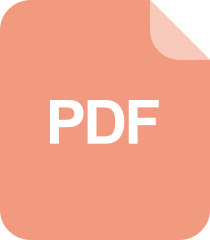
android实现点击按钮切换不同的fragment布局
在Android中,你可以使用Fragment来创建自定义界面,并结合四个Button来实现点击按钮时背景颜色的变化。首先,你需要在Fragment布局文件中添加四个Button。然后,为每个Button设置一个监听器,当按钮被点击时,会触发一个方法更改其背景颜色。
以下是基本步骤:
1. **在XML布局文件** (activity_main.xml 或 fragment_layout.xml) 中添加四个Button,例如:
```xml
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 1"
/>
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 2"
/>
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 3"
/>
<Button
android:id="@+id/button4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 4"
/>
```
2. **在Fragment内部** (MyFragment.java 或 MainActivity.java),给每个Button设置OnClickListener:
```java
public class MyFragment extends Fragment {
private Button button1, button2, button3, button4;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_layout, container, false);
button1 = view.findViewById(R.id.button1);
button2 = view.findViewById(R.id.button2);
button3 = view.findViewById(R.id.button3);
button4 = view.findViewById(R.id.button4);
// 设置点击事件监听
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
changeBackgroundColor(v.getId());
}
});
// 添加其他三个按钮的监听器,代码类似
button2.setOnClickListener(...);
button3.setOnClickListener(...);
button4.setOnClickListener(...);
return view;
}
// 改变按钮背景颜色的通用方法
private void changeBackgroundColor(int buttonId) {
switch (buttonId) {
case R.id.button1:
button1.setBackgroundColor(Color.RED); // 示例:设置红色
break;
case R.id.button2:
button2.setBackgroundColor(Color.GREEN);
break;
case R.id.button3:
button3.setBackgroundColor(Color.BLUE);
break;
case R.id.button4:
button4.setBackgroundColor(Color.YELLOW);
break;
}
}
}
```
阅读全文
相关推荐
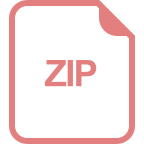
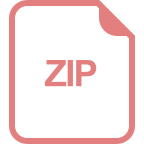

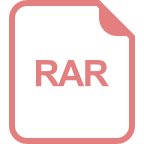
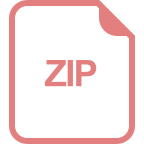
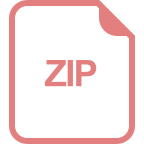
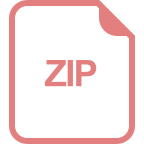
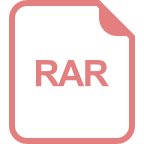


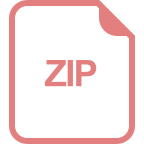
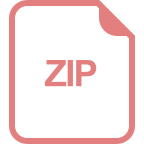
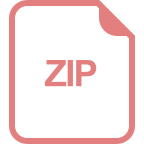
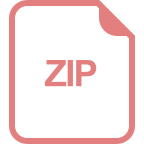
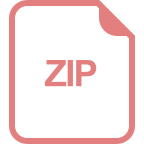
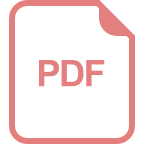
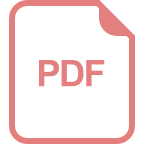
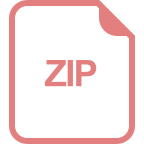
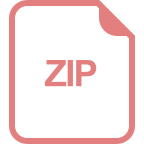