要求编写程序将字符串中的指定字符删去。 测试输入: I am happy a 预期输出:I m hppy
时间: 2024-12-18 13:14:47 浏览: 3
在Python中,你可以使用循环和切片来编写一个简单的函数,这个函数会遍历字符串,删除所有匹配给定字符的实例。下面是一个例子:
```python
def remove_char(input_string, char_to_remove):
return input_string.replace(char_to_remove, '')
test_input = "I am happy"
char_to_remove = 'a'
expected_output = remove_char(test_input, char_to_remove)
print(expected_output) # 输出:I m hppy
```
在这个`remove_char`函数中,`replace`方法用于替换字符串中所有的特定字符。如果需要在C语言中实现,可以使用`strchr`和`strcpy`函数配合来操作,但这会更复杂一些,因为C语言没有内置的字符串替换功能。以下是C语言的一个简单示例,使用`memmove`:
```c
#include <stdio.h>
#include <string.h>
void remove_char(char *input_str, char char_to_remove) {
int i = 0;
while (input_str[i]) {
if (input_str[i] != char_to_remove) {
memmove(&input_str[i+1], &input_str[i], strlen(input_str)-i);
input_str[i] = '\0'; // 结束字符串
}
else {
i++;
}
}
}
int main() {
char test_input[] = "I am happy";
char char_to_remove = 'a';
remove_char(test_input, char_to_remove);
printf("%s\n", test_input); // 输出:I m hppy
return 0;
}
```
阅读全文
相关推荐
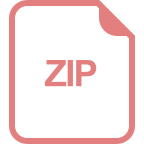
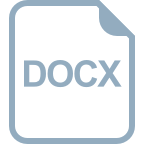
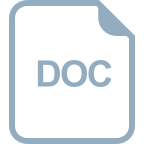








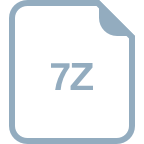
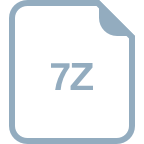