双倍体遗传算法代码实例
时间: 2024-10-24 14:01:08 浏览: 22
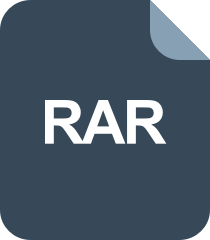
常用的7种C#遗传算法源码实例集
双倍体遗传算法是一种基于自然选择、交叉和变异等生物进化原理的优化搜索算法。它通常用于解决复杂的全局优化问题,比如函数优化、机器学习模型参数调整等。这里是一个简单的Python代码示例,使用DEAP库(Distributed Evolutionary Algorithms in Python)实现:
```python
from deap import base, creator, tools
# 定义染色体类型
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
def mate(ind1, ind2):
return [ind1[i] + ind2[i] for i in range(len(ind1))]
def mutate(individual):
individual[random.randint(0, len(individual) - 1)] += random.randint(-1, 1)
return individual
toolbox = base.Toolbox()
toolbox.register("individual", creator.Individual, range(10)) # 假设我们有一个10维的搜索空间
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("mate", mate)
toolbox.register("mutate", mutate)
toolbox.register("select", tools.selTournament, tournsize=3)
toolbox.register("evaluate", evaluate_function) # 自定义的目标函数
def evolve(population,ngen=50, cxpb=0.5, mutpb=0.2):
pop = toolbox.population(n=population)
hof = tools.HallOfFame(1)
for g in range(ngen):
offspring = [toolbox.clone(ind) for ind in pop]
# 遗传操作
for _ in range(int(len(offspring)/2)):
if random.random() < cxpb:
offspring[random.randint(0, len(offspring)-1)], offspring[random.randint(0, len(offspring)-1)] = toolbox.mate(offspring[random.randint(0, len(offspring)-1)], offspring[random.randint(0, len(offspring)-1)])
offspring[random.randint(0, len(offspring)-1)].fitness.values = toolbox.evaluate(offspring[random.randint(0, len(offspring)-1)])
# 变异操作
for ind in offspring:
if random.random() < mutpb:
toolbox.mutate(ind)
ind.fitness.values = toolbox.evaluate(ind)
# 更新种群并保存最佳解
population[:] = toolbox.select(offspring, k=len(pop))
hof.update(pop)
return pop, hof
best_solution, best_fitness = evolve(100)
print(f"Best solution found: {best_solution}, Fitness: {best_fitness[0].fitness}")
阅读全文
相关推荐
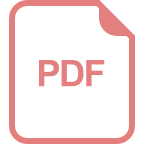
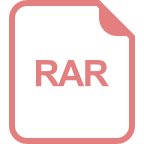






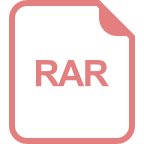

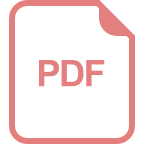
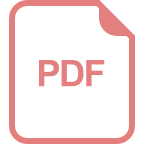
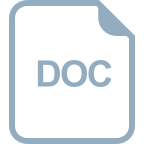
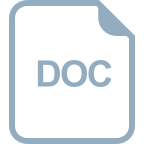
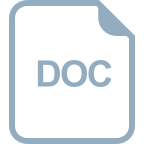
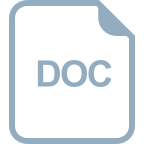

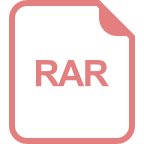
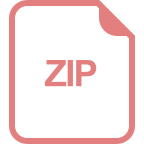