python爬虫快速入门
时间: 2024-08-08 09:01:44 浏览: 83
Python 爬虫快速入门:
### 一、安装必要的库
首先,你需要安装 Python 的一些基本库用于构建爬虫。
1. **BeautifulSoup**: 用于解析 HTML 和 XML 文档,并提取所需信息。
```bash
pip install beautifulsoup4
```
2. **requests**: 发送 HTTP 请求获取网页内容。
```bash
pip install requests
```
3. **Pandas**: 数据处理工具,可以方便地存储和操作数据。
```bash
pip install pandas
```
4. **Scrapy**: 一款强大的 Web 挖掘框架,可以自动化抓取数据。
```bash
pip install scrapy
```
### 二、编写基础爬虫代码
#### 使用 BeautifulSoup 和 Requests
```python
from bs4 import BeautifulSoup
import requests
def scrape_web(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
# 找到页面中某个特定标签的内容,这里只是示例,实际应用会根据网站结构调整
for link in soup.find_all('a'):
print(link.get('href'))
else:
print(f"Failed to retrieve the web page. Status code: {response.status_code}")
url_to_scrape = "https://example.com"
scrape_web(url_to_scrape)
```
#### 使用 Scrapy
创建项目并添加 spider:
```bash
scrapy startproject mycrawler
cd mycrawler
scrapy genspider example "example.com"
```
编辑 `mycrawler/example/spiders/example.py`:
```python
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
allowed_domains = ['example.com']
start_urls = ['http://example.com/']
def parse(self, response):
for link in response.css('a::attr(href)'):
yield {'link': link.extract()}
# Follow links to crawl deeper into the site.
for href in response.css('div.next a::attr(href)').extract():
url = response.urljoin(href)
yield scrapy.Request(url=url, callback=self.parse)
```
运行爬虫:
```bash
scrapy crawl example
```
### 三、处理数据和存储
利用 Pandas 将收集的数据整理和保存成 CSV 文件或其他格式:
```python
import pandas as pd
data = [
{"name": "John Doe", "age": 30},
{"name": "Jane Smith", "age": 25}
]
df = pd.DataFrame(data)
df.to_csv("output.csv", index=False)
```
### 四、遵守法律法规及网站条款
在进行网络爬虫活动前务必了解目标网站的 robots.txt 文件以及其服务条款,避免违反法律和网站政策。
---
相关推荐
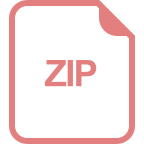
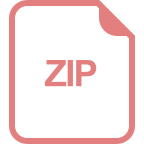
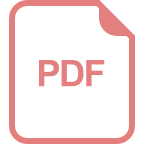
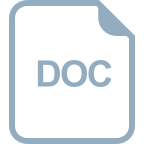
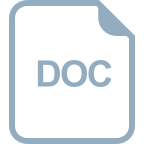
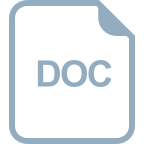
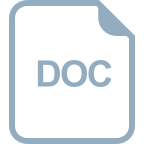
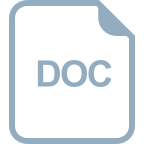
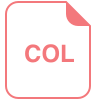
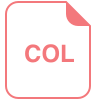
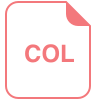
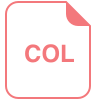
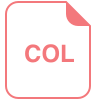




