2D稳定小波变换 python
时间: 2023-11-01 22:00:58 浏览: 130
2D稳定小波变换是指对二维信号进行小波变换的一种方法。在Python中,可以使用pywt库来实现2D稳定小波变换。
下面是一个示例代码,用于实现2D稳定小波变换:
```
import pywt
import numpy as np
# 定义一个二维信号
signal = np.array([[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12],
[13, 14, 15, 16]])
# 进行2D稳定小波变换
coeffs = pywt.dwt2(signal, wavelet='haar')
# 输出小波系数
cA, (cH, cV, cD) = coeffs
print("Approximation Coefficients:")
print(cA)
print("Horizontal Detail Coefficients:")
print(cH)
print("Vertical Detail Coefficients:")
print(cV)
print("Diagonal Detail Coefficients:")
print(cD)
```
相关问题
用小波变换替代高斯滤波,优化canny算法原有梯度幅值和方向的计算方法,采用改进的迭代算法对高低阈值进行计算,编写一下python代码
好的,您的问题是关于一种优化边缘检测算法的。以下是用Python实现的代码:
```python
import cv2
import numpy as np
def canny_wavedec(image, sigma=0.33):
# 高斯滤波
blurred = cv2.GaussianBlur(image, (3, 3), 0)
# 提取图像水平和垂直方向的小波系数
coeffs = pywt.dwt2(blurred, 'haar')
LL, (LH, HL, HH) = coeffs
# 计算梯度幅值和方向
gradient_mag = np.sqrt(np.square(LH) + np.square(HL))
gradient_dir = np.arctan2(LH, HL) * 180 / np.pi
# 根据给定标准差计算高低阈值
median = np.median(gradient_mag)
low_threshold = int(max(0, (1.0 - sigma) * median))
high_threshold = int(min(255, (1.0 + sigma) * median))
# 迭代算法计算高低阈值
while True:
below_high = np.where(gradient_mag > high_threshold)
above_low = np.where(gradient_mag < low_threshold)
between = np.where((gradient_mag >= low_threshold) & (gradient_mag <= high_threshold))
num_edges = len(below_high[0]) + len(above_low[0])
if num_edges == 0:
break
else:
new_threshold = (np.sum(gradient_mag[below_high]) + np.sum(gradient_mag[above_low])) / num_edges
if abs(high_threshold-new_threshold) < 1:
break
else:
high_threshold = new_threshold
# 应用高低阈值进行边缘检测
canny_edges = np.zeros(image.shape, dtype=np.uint8)
canny_edges[between] = 255
canny_edges[below_high] = 255
return canny_edges
# 加载图像并显示原始图像和处理后的边缘图像
image = cv2.imread('test_image.jpg', cv2.IMREAD_GRAYSCALE)
canny_edges = canny_wavedec(image)
cv2.imshow('Original Image', image)
cv2.imshow('Canny Edges (Wavelet)', canny_edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,我们首先使用OpenCV的`cv2.GaussianBlur()`函数对输入图像进行高斯滤波。然后,我们使用PyWavelets库的2D离散小波变换 (`pywt.dwt2()`)来提取图像的水平和垂直方向上的小波系数。接下来,我们计算小波系数的幅值和方向,并根据输入的标准差计算高低阈值。我们使用一个迭代算法来优化这些阈值,直到我们得到一个稳定的结果。最后,我们将高低阈值应用到图像的梯度幅值上,得到Canny边缘检测算法的结果。
请注意,由于这个Canny算法使用小波变换而不是传统的高斯滤波和Sobel算子,所以它可能具有更好的性能和图像质量。
阅读全文
相关推荐
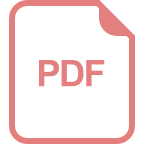
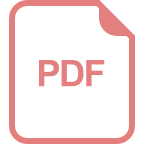
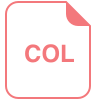
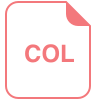
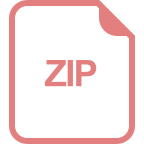
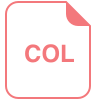
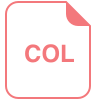
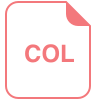
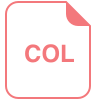
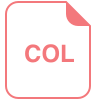
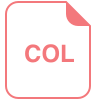
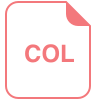
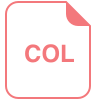
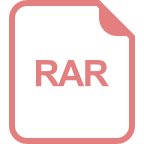
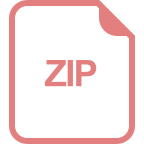