WebDriver.find_element() takes from 1 to 3 positional arguments but 4 were given
时间: 2024-06-22 22:02:21 浏览: 9
`WebDriver.find_element()` 是一个方法,通常用于Web自动化测试框架 Selenium 中,用于查找网页上的元素。这个方法通常接受一到三个位置参数,比如元素的定位器(如 ID、CSS选择器、XPath 或其他类型),可能还会接收一个可选的超时参数来设置查找元素的等待时间。
当调用该方法时,如果你提供了超过它定义的最大参数数量,例如传递了四个参数,那么会抛出 `TypeError`,因为方法的签名只接受1到3个参数,而你传递了超出范围的值。这可能是由于以下原因:
1. 你可能误将额外的参数(如默认超时或其他非预期参数)添加到了方法调用中。
2. 参数顺序错误,如果文档中有默认值,你可能无意中按照默认值的顺序提供了过多的参数。
正确的调用应该是这样:
```python
element = driver.find_element(locator, timeout=your_timeout_value) # 如果需要设置超时
```
如果遇到错误,请检查文档以确认正确参数的使用方式,并确保提供的参数数量符合方法的预期。
相关问题
move_to_element() takes 2 positional arguments but 3 were given
这个错误提示通常是由于调用 `move_to_element()` 方法时传递了多余的参数导致的。`move_to_element()` 方法只接受一个参数,即要移动到的元素对象,而不接受其他参数。因此,如果在调用该方法时传递了多余的参数,就会出现类似于 "move_to_element() takes 2 positional arguments but 3 were given" 的错误提示。
例如,以下代码就会引发上述错误:
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
# 创建 WebDriver 对象
driver = webdriver.Chrome()
# 打开网页
driver.get('https://www.example.com')
# 定位要移动到的元素
element = driver.find_element_by_xpath('//a[@class="example"]')
# 创建 ActionChains 对象
actions = ActionChains(driver)
# 将鼠标移动到元素位置,并指定偏移量
actions.move_to_element(element, 10, 10).perform()
```
在上面的代码中,`move_to_element()` 方法被传递了三个参数:要移动到的元素对象 `element`,以及 x 和 y 方向上的偏移量。然而,`move_to_element()` 方法只接受一个参数,因此会出现类似于 "move_to_element() takes 2 positional arguments but 3 were given" 的错误提示。
要修复这个错误,可以删除多余的参数,只传递要移动到的元素对象即可。例如:
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
# 创建 WebDriver 对象
driver = webdriver.Chrome()
# 打开网页
driver.get('https://www.example.com')
# 定位要移动到的元素
element = driver.find_element_by_xpath('//a[@class="example"]')
# 创建 ActionChains 对象
actions = ActionChains(driver)
# 将鼠标移动到元素位置
actions.move_to_element(element).perform()
```
在上面的代码中,只传递了要移动到的元素对象 `element`,没有传递其他参数,因此可以避免出现 "move_to_element() takes 2 positional arguments but 3 were given" 的错误提示。
from selenium.webdriver.common.by import By ele = self.find_element(By.XPATH, '//*[@id="app"]/div/div[2]/section/div/div[1]/div/div[3]/div[1]') 报错:TypeError: find_element() takes 2 positional arguments but 3 were given
这个报错可能是因为你在调用 `find_element` 方法时,将 `By.XPATH` 和选择器字符串作为两个独立的参数传递给了该方法,而 `find_element` 方法只接受一个参数,即一个元组类型的 `locator`。
你可以尝试修改代码,将 `By.XPATH` 和选择器字符串作为一个元组传递给 `find_element` 方法,例如:
```
from selenium.webdriver.common.by import By
ele = self.find_element((By.XPATH, '//*[@id="app"]/div/div[2]/section/div/div[1]/div/div[3]/div[1]'))
```
这样就可以避免这个报错了。
相关推荐
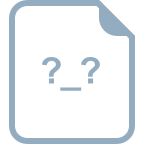
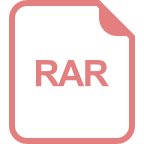
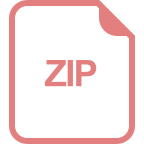













