实现一个双向链表的插入接口
时间: 2024-09-14 11:09:26 浏览: 42
实现一个双向链表的插入操作通常涉及到以下几个步骤:
1. **创建节点**:首先,你需要定义一个节点类,包含两个属性,一个是存储数据的值,另一个是指向下一个节点的指针(称为`next`),还有一个指向前一个节点的指针(称为`prev`)。在Python中,可以这样定义:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
self.prev = None
```
2. **头结点处理**:如果链表为空,新节点将成为头结点。这时,它的`prev`应设置为None。
3. **常规插入**:对于非头结点的插入,你需要找到插入位置的前一个节点,并将新节点的`prev`指向该节点,新节点的`next`指向原节点,然后更新原节点的`prev`指针。
```python
def insert(self, data, position):
new_node = Node(data)
if position == 0: # 插入到头部
if self.head is not None:
new_node.next = self.head
self.head.prev = new_node
self.head = new_node
else:
current = self.head
index = 0
while current and index < position - 1:
current = current.next
index += 1
if current:
new_node.prev = current
new_node.next = current.next
if current.next:
current.next.prev = new_node
current.next = new_node
```
4. **尾部插入**:当需要在链表末尾插入时,只需让新节点的`next`指向当前尾部节点,并更新尾部节点的`next`。
5. **验证**:记得在插入之后检查并维护双向链表的结构完整性。
阅读全文
相关推荐




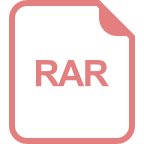
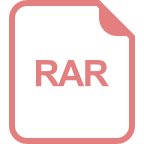
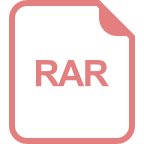











