android 读取RAW中的bmp图片为RGBA类型byte数组
时间: 2024-12-16 13:29:00 浏览: 5
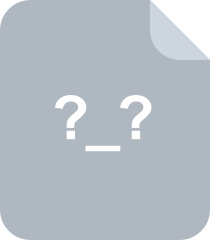
RGBA数据转存BMP图片
在Android中,如果你想要从Raw资源文件(通常存储在res/raw目录下的.bmp图像文件)读取并转换为RGB或RGBA类型的字节数组,可以按照以下步骤操作:
1. 首先,你需要在AndroidManifest.xml文件中声明该资源文件:
```xml
<resources>
<raw name="image_bmp" type="image/bmp" />
</resources>
```
2. 然后,在需要读取图片的地方,创建一个Bitmap对象,通过ContentResolver获取到资源流:
```java
InputStream is = context.getResources().openRawResource(R.raw.image_bmp);
```
3. 使用` BitmapFactory`加载这个输入流,生成Bitmap:
```java
BitmapFactory.Options options = new BitmapFactory.Options();
options.inPreferredConfig = Bitmap.Config.RGB_565; // 或者Bitmap.Config.ARGB_8888取决于需求
Bitmap bitmap = BitmapFactory.decodeStream(is, null, options);
```
4. 如果你想得到RGBA类型的字节数组,需要将Bitmap转化为字节数组,并手动处理像素数据:
```java
byte[] pixelData;
if (bitmap.getConfig() == Bitmap.Config.RGB_565) {
int width = bitmap.getWidth();
int height = bitmap.getHeight();
pixelData = new byte[width * height * 4]; // 因为RGBA有四个通道
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int pixel = bitmap.getPixel(x, y);
int alpha = (pixel >> 16) & 0xFF; // 取出alpha通道
int red = (pixel >> 11) & 0x1F; // 取出red通道
int green = (pixel >> 5) & 0x3F; // 取出green通道
int blue = pixel & 0x1F; // 取出blue通道
pixelData[(y * width + x) * 4] = (byte) blue; // R
pixelData[(y * width + x) * 4 + 1] = (byte) green; // G
pixelData[(y * width + x) * 4 + 2] = (byte) red; // B
pixelData[(y * width + x) * 4 + 3] = (byte) alpha; // A
}
}
} else if (bitmap.getConfig() == Bitmap.Config.ARGB_8888) {
// 如果已经是ARGB,直接复制数据即可
pixelData = bitmap.copy(Bitmap.Config.ARGB_8888, false).getPixels();
}
```
阅读全文
相关推荐
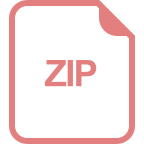
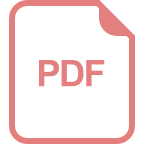















